This tutorial describes how to integrate update functionality into Eclipse RCP applications using Eclipse p2.
1. Prerequisites
This tutorial assumes that you have a basic understanding of development for the Eclipse platform.
Please see https://www.vogella.com/tutorials/EclipseRCP/article.html and https://www.vogella.com/tutorials/EclipsePlugin/article.html for an introduction. It also assumes that you know how to create Eclipse feature projects.
2. Eclipse application updates with p2
2.1. Eclipse application updates
The Eclipse platform provides an installation and update mechanism for Eclipse applications. This mechanism is called Eclipse p2 (short: p2).
The update and installation of functions with p2 is typical based on feature projects (short: features). It is possible to update complete products or individual features. In the terminology of p2 these features are installable units.
2.2. Creating p2 update sites
Installable units can be grouped into a p2 repository with is a predefined set of files. An update site contains files for describing artifacts and metadata.
Typically, these files are copied to a web server so users can install new functionality or upgrades using the p2 mechanism. A p2 repository is also called update site.
p2 can refer to a repository via an URI which can point to a local file system or to a web server.
If you build an Eclipse application, you also create an update site. This can for example be done with Maven Tycho.
2.3. Manual product export with p2 update site
For example, if you export it from the user interface you can select the Generate p2 repository option in the Eclipse product export dialog. This option is highlighted in the following screenshot.
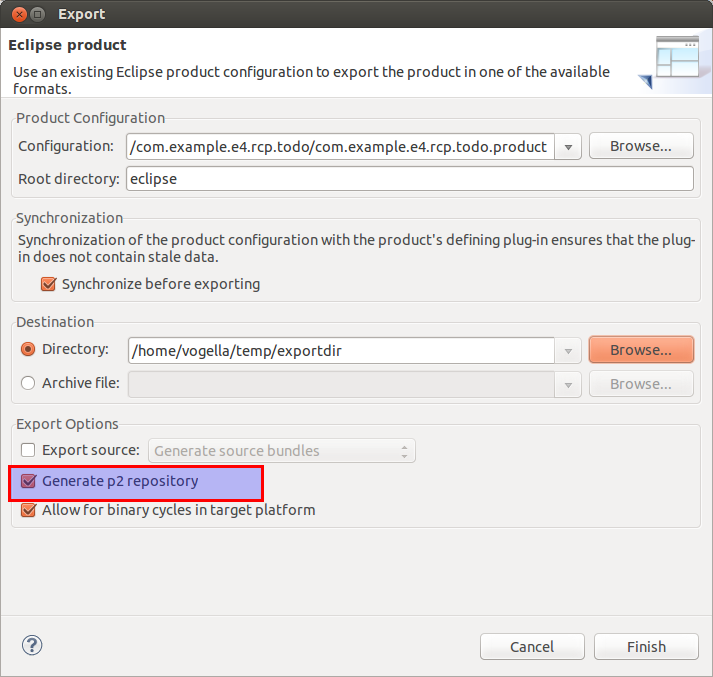
If you select this option, an update site is created in a sub-folder called repository of the export directory.
3. Required plug-ins for updates
The following table lists the core plug-ins and the features, which provide the non-user interface functionality of p2.
Plug-in | Description |
---|---|
org.eclipse.equinox.p2.core |
Core p2 functionality. |
org.eclipse.equinox.p2.engine |
The engine carries out the provisioning operation. |
org.eclipse.equinox.p2.operations |
Layer over the core and engine API to describe updates as an atomic install. |
org.eclipse.equinox.p2.metadata.repository |
Contains the definition of p2 repositories. |
org.eclipse.equinox.p2.core.feature |
Feature containing the p2 bundles. |
To use the Eclipse update API you need to include these plug-ins as dependencies to your manifest file. And you must add the feature to your product configuration file.
When the Eclipse installation is used as target platform, these required plugins should be already available. Otherwise they can be installed or added to a target definition file like this:
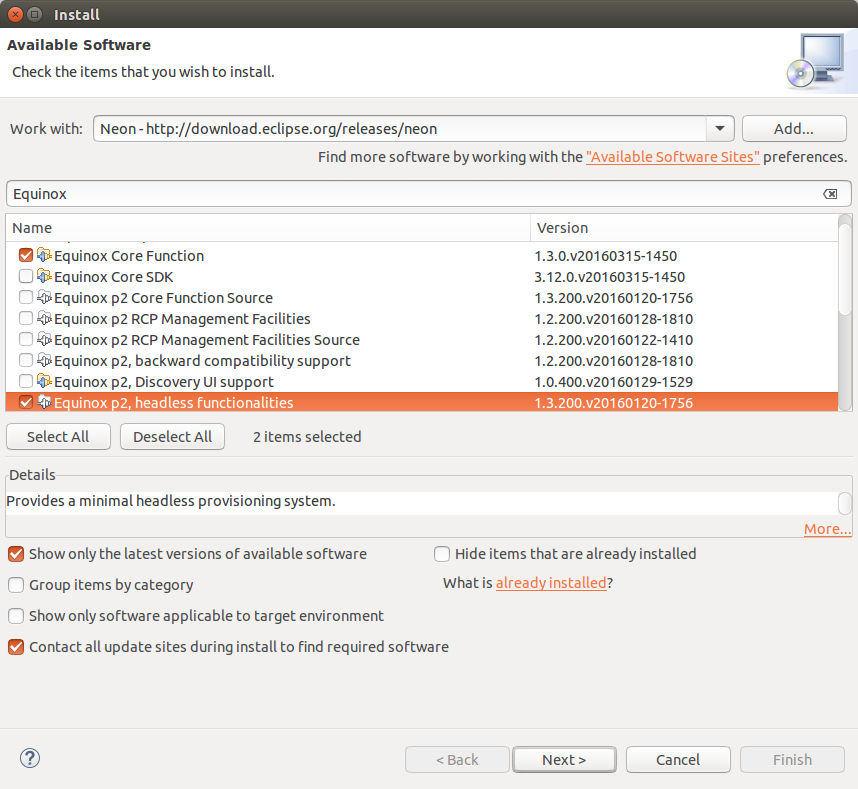
4. Updating Eclipse RCP applications
To update your Eclipse 4 application you use the p2 API. This is demonstrates in the tutorial.
6. Adding p2 update sites
If you place a p2.inf file beside your product configuration file you can add update sites to your product. The following listing is an example for that.
instructions.configure=\
addRepository(type:0,location:http${#58}//download.eclipse.org/eclipse/updates/4.5,name:Eclipse Mars Update Site);\
addRepository(type:1,location:http${#58}//download.eclipse.org/eclipse/updates/4.5,name:Eclipse Mars Update Site);
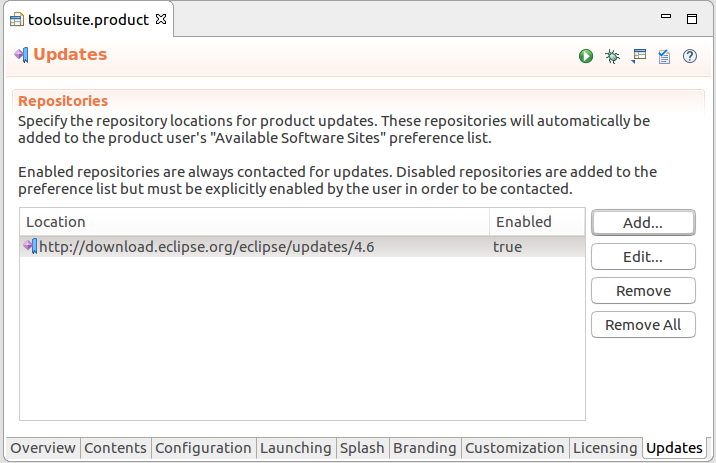
7. Custom install actions (touchpoints) in p2
The Eclipse p2 functionality invokes actions (touchpoints) when an installable unit is installed, configured, or uninstalled. For example, it is possible to unzip or copy artifacts or to set file permissions.
The Eclipse p2 API uses install actions for this, called touchpoints. By default, it supports two touchpoint types, native and OSGi types. Native touchpoint actions are not directly related to Eclipse (such as file commands) while the OSGi ones are Eclipse specific.
It is possible to add custom touchpoint actions via the org.eclipse.equinox.p2.engine.action extension point. The class which implements this touchpoint action must implement ProvisioningAction.
p2 must be instructed to run this touchpoint. For this, you need to specify the action as requirement via a MetaRequirements section in the META-INF/p2.inf file of the corresponding plug-in. It must also define how to invoke the execution of the custom touchpoint.
See http://eclipsesource.com/blogs/2013/05/23/custom-touchpoints-in-p2 for a detailed example.
8. p2 composite repositories
It is possible to create p2 composite repositories. Such a repository wraps the information about other repositories. If a client connects to such an update site, the other update sites are contacted and the user can install features from the other update site.
To build such a composite repository you have to create two files. The first file must be called compositeContent.xml. The second file must be called compositeArtifacts.xml. The following example demonstrates the content of these files.
The following listing is an example for an compositeContent.xml file.
<?xml version='1.0' encoding='UTF-8'?>
<?compositeMetadataRepository version='1.0.0'?>
<repository name='"oclipse development environment"'
type='org.eclipse.equinox.internal.p2.metadata.repository.CompositeMetadataRepository'
version='1.0.0'>
<properties size='1'>
<property name='p2.timestamp' value='1243822502499' />
</properties>
<children size='2'>
<child location='https://download.eclipse.org/releases/luna/'/>
<child location='http://dl.bintray.com/vogellacompany/eclipse-preference-spy/'/>
</children>
</repository>
The following listing is an example for a fitting compositeArtifacts.xml file.
<?xml version='1.0' encoding='UTF-8'?>
<?compositeArtifactRepository version='1.0.0'?>
<repository name='"voclipse development environment"'
type='org.eclipse.equinox.internal.p2.artifact.repository.CompositeArtifactRepository'
version='1.0.0'>
<properties size='1'>
<property name='p2.timestamp' value='1243822502440' />
</properties>
<children size='2'>
<child location='https://download.eclipse.org/releases/luna/' />
<child location='http://dl.bintray.com/vogellacompany/eclipse-preference-spy/' />
</children>
</repository>
9. Eclipse p2 update resources
https://wiki.eclipse.org/Equinox_p2_Repository_Mirroring Mirroring a p2 repository to a local side
If you need more assistance we offer Online Training and Onsite training as well as consulting