Apache Ant Tutorial. This tutorial describes the usage of Ant as a build tool to compile Java code, pack this code into an executable jar and how to create Javadoc. The usage of Ant is demonstrated within Eclipse and from the command line. This tutorial is based on Apache Ant 1.8.x.
1. Build tools
In software development the term building usually means the conversion of source code and other artifacts, like images or configuration files, into another artifact. For example, source code might be compiled into a JAR file, or you may create a new standalone application. The build result can be shared with users of the software or used only internally.
A build tool is used to automate repetitive tasks during this process. This can be, for example, compiling source code, running software tests and creating files and documentation for the software deployment.
Build tools typically run without a graphical user interface directly from the command line. As a user interface is not required for such builds, these builds are called headless.
Popular build tools in the Java space are Maven, Gradle and Apache Ant.
2. Apache Ant Overview
2.1. What is Apache Ant?
Apache Ant (Ant) is a general purpose build tool. Ant is an abbreviation for Another Neat Tool.
Ant is primarily used for building and deploying Java projects but can be used for every possible repetitive tasks, e.g. generating documentation.
2.2. Configuring Apache Ant
A Java build process typically includes:
-
the compilation of the Java source code into Java bytecode
-
creation of the .jar file for the distribution of the code
-
creation of the Javadoc documentation
Ant uses an xml
file for its configuration.
The default file name is build.xml
.
Ant builds are based on three blocks: tasks, targets and extension points.
A task is a unit of work which should be performed and constitutes of small atomic steps, for example compile source code or create Javadoc. Tasks can be grouped into targets.
A target can be directly invoked via Ant. Targets can specify their dependencies. Ant will automatically execute all dependent targets.
For example, if target A depends on B and Ant is instructed to run A, it first runs B before running A.
In your build.xml
file you can specify the default target.
Ant executes this target, if no explicit target is specified.
3. Installation
3.1. Prerequisites
Ant requires the installation of the Java Development Kit (JDK). See Java introduction for a description how to install Java.
3.2. Ubuntu
On Ubuntu use the apt-get install ant
command to install Ant.
For other distributions please check the documentation of your vendor.
3.3. Windows
Download Apache Ant from http://ant.apache.org/.
Extract the zip file into a directory structure of your choice.
Set the ANT_HOME
environment variable to this location and include the ANT_HOME/bin
directory in your path.
Make also sure that the JAVA_HOME environment variable is set to the JDK. This is required for running Ant.
Check your installation by opening a command line and typing ant -version
into the commend line.
The system should find the command ant and show the version number of your installed Ant version.
4. Tutorial: Using Apache Ant
4.1. Using Ant for Java development
The following describes how you compile Java classes, create an executable JAR file and create Javadoc for your project with Apache Ant. The following example assumes that you are using the Eclipse IDE to manage your Java project and your Ant build.
4.2. Create Java project
Create a Java project called de.vogella.build.ant.first in Eclipse. Create a package called math and the following class.
package math;
public class MyMath {
public int multi(int number1, int number2) {
return number1 * number2;
}
}
Create the test
package and the following class.
package test;
import math.MyMath;
public class Main {
public static void main(String[] args) {
MyMath math = new MyMath();
System.out.println("Result is: " + math.multi(5, 10));
}
}
4.3. Create build.xml
Create a new file through the build.xml
file.
Implement the following code to this file.
<?xml version="1.0"?>
<project name="Ant-Test" default="main" basedir=".">
<!-- Sets variables which can later be used. -->
<!-- The value of a property is accessed via ${} -->
<property name="src.dir" location="src" />
<property name="build.dir" location="bin" />
<property name="dist.dir" location="dist" />
<property name="docs.dir" location="docs" />
<!-- Deletes the existing build, docs and dist directory-->
<target name="clean">
<delete dir="${build.dir}" />
<delete dir="${docs.dir}" />
<delete dir="${dist.dir}" />
</target>
<!-- Creates the build, docs and dist directory-->
<target name="makedir">
<mkdir dir="${build.dir}" />
<mkdir dir="${docs.dir}" />
<mkdir dir="${dist.dir}" />
</target>
<!-- Compiles the java code (including the usage of library for JUnit -->
<target name="compile" depends="clean, makedir">
<javac srcdir="${src.dir}" destdir="${build.dir}">
</javac>
</target>
<!-- Creates Javadoc -->
<target name="docs" depends="compile">
<javadoc packagenames="src" sourcepath="${src.dir}" destdir="${docs.dir}">
<!-- Define which files / directory should get included, we include all -->
<fileset dir="${src.dir}">
<include name="**" />
</fileset>
</javadoc>
</target>
<!--Creates the deployable jar file -->
<target name="jar" depends="compile">
<jar destfile="${dist.dir}\de.vogella.build.test.ant.jar" basedir="${build.dir}">
<manifest>
<attribute name="Main-Class" value="test.Main" />
</manifest>
</jar>
</target>
<target name="main" depends="compile, jar, docs">
<description>Main target</description>
</target>
</project>
The code is documented, you should be able to determine the purpose of the different ant tasks via the documentation in the coding.
4.4. Run your Ant build from Eclipse
Run the build.xml
file as an Ant Build in Eclipse.
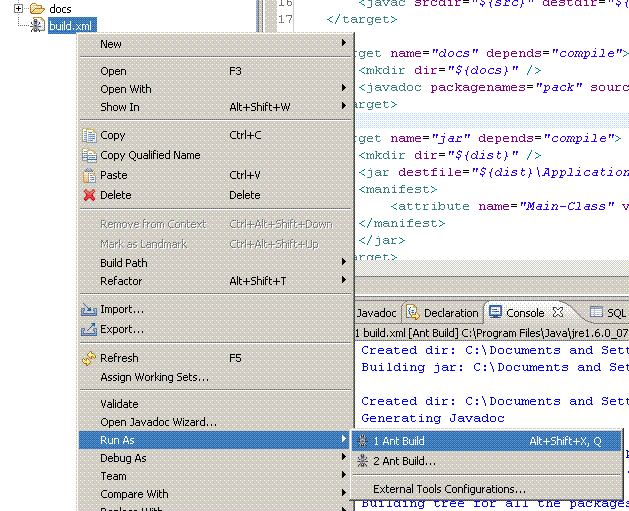
After this process your data structure should look like this:
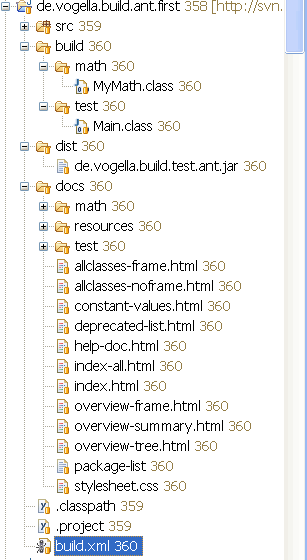
4.5. Run your Ant build from the command line
Open a command line and switch to your project directory. Type the following commands.
# run the build
ant -f build.xml
# run ant with defaults (build.xml file and the default target)
ant
The build should finish successfully and generate the build artifacts.
5. Apache Ant classpath
5.1. Setting the Ant classpath
Ant allows to create classpath containers and use them in tasks.
The following build.xml
from a project called de.vogella.build.ant.classpath demonstrates this.
<?xml version="1.0"?>
<project name="Ant-Test" default="Main" basedir=".">
<!-- Sets variables which can later be used. -->
<!-- The value of a property is accessed via ${} -->
<property name="src.dir" location="src" />
<property name="lib.dir" location="lib" />
<property name="build.dir" location="bin" />
<!--
Create a classpath container which can be later used in the ant task
-->
<path id="build.classpath">
<fileset dir="${lib.dir}">
<include name="**/*.jar" />
</fileset>
</path>
<!-- Deletes the existing build directory-->
<target name="clean">
<delete dir="${build.dir}" />
</target>
<!-- Creates the build directory-->
<target name="makedir">
<mkdir dir="${build.dir}" />
</target>
<!-- Compiles the java code -->
<target name="compile" depends="clean, makedir">
<javac srcdir="${src.dir}" destdir="${build.dir}" classpathref="build.classpath" />
</target>
<target name="Main" depends="compile">
<description>Main target</description>
</target>
</project>
5.2. Print
You can use the following statements to write the full classpath to the console. You can use this to easily verify if the classpath is correct.
<!-- Write the classpath to the console. Helpful for debugging -->
<!-- Create one line per classpath element-->
<pathconvert pathsep="${line.separator}" property="echo.classpath" refid="junit.class.path">
</pathconvert>
<!-- Write the result to the console -->
<echo message="The following classpath is associated with junit.class.path " />
<echo message="${echo.classpath}" />
6. Run Java program
Ant allows to run Java programs. You can specify properties which are passed as parameters to the main method of Java. The following demonstrates how to run a Java program.
<project name="Run java program" basedir="." default="default">
<property name="outputdir.property" value="../../output/"/>
<property name="inputdir.property" value="../../output/"/>
<property name="classpath.property" value="${basedir}/../com.vogella.website.repository/target/repository/plugins/"/>
<target name="default">
<path id="runtime.path">
<fileset dir="${classpath.property}" includes="*.jar"/>
</path>
<echo message = "Ant output directory: ${outputdir.property}"/>
<echo message = "Ant input directory for tutorials: ${inputdir.property}"/>
<echo message = "Ant classpath directory: ${classpath.property}"/>
<java classname="com.vogella.test.Mail" fork="false" classpathref="runtime.path">
<arg value="${outputdir.property}"/>
<arg value="${inputdir.property}"/>
</java>
</target>
</project>
7. Running JUnit Tests via Ant
Ant allows to run JUnit tests. Ant defines junit task. You only need to include the junit.jar and the compiled classes into the classpath for Ant and then you can run JUnit tests. See JUnit Tutorial for an introduction into JUnit.
The following is a JUnit test for the previous example.
package test;
import math.MyMath;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyMathTest {
@Test
public void testMulti() {
MyMath math = new MyMath();
assertEquals(50, math.multi(5, 10));
}
}
You can run this JUnit unit test via the following build.xml. This example assumes that the JUnit jar "junit.jar" is in folder "lib".
<?xml version="1.0"?>
<project name="Ant-Test" default="main" basedir=".">
<!-- Sets variables which can later be used. -->
<!-- The value of a property is accessed via ${} -->
<property name="src.dir" location="src" />
<property name="build.dir" location="bin" />
<!-- Variables used for JUnit testin -->
<property name="test.dir" location="src" />
<property name="test.report.dir" location="testreport" />
<!-- Define the classpath which includes the junit.jar and the classes after compiling-->
<path id="junit.class.path">
<pathelement location="lib/junit.jar" />
<pathelement location="${build.dir}" />
</path>
<!-- Deletes the existing build, docs and dist directory-->
<target name="clean">
<delete dir="${build.dir}" />
<delete dir="${test.report.dir}" />
</target>
<!-- Creates the build, docs and dist directory-->
<target name="makedir">
<mkdir dir="${build.dir}" />
<mkdir dir="${test.report.dir}" />
</target>
<!-- Compiles the java code (including the usage of library for JUnit -->
<target name="compile" depends="clean, makedir">
<javac srcdir="${src.dir}" destdir="${build.dir}">
<classpath refid="junit.class.path" />
</javac>
</target>
<!-- Run the JUnit Tests -->
<!-- Output is XML, could also be plain-->
<target name="junit" depends="compile">
<junit printsummary="on" fork="true" haltonfailure="yes">
<classpath refid="junit.class.path" />
<formatter type="xml" />
<batchtest todir="${test.report.dir}">
<fileset dir="${src.dir}">
<include name="**/*Test*.java" />
</fileset>
</batchtest>
</junit>
</target>
<target name="main" depends="compile, junit">
<description>Main target</description>
</target>
</project>
8. Useful Ant tasks
8.1. fail
Ant provides the fail
task which allows to fail the build if a condition is not met.
For example, the following task would check for the existence of a file and if this file is not present the build would fail.
<fail message="PDF file was not created.">
<condition>
<not>
<available file="${pdf.dir}/path/book.pdf" />
</not>
</condition>
</fail>
Or you can check for the number of files generated.
<fail message="Files are missing.">
<condition>
<not>
<resourcecount count="2">
<fileset id="fs" dir="." includes="one.txt,two.txt"/>
</resourcecount>
</not>
</condition>
</fail>
9. Eclipse Ant Editor
Eclipse has an ant editor which makes the editing of ant files very easy by providing syntax checking of the build file. Eclipse has also an ant view. In this view you execute ant files via double-clicking on the target.
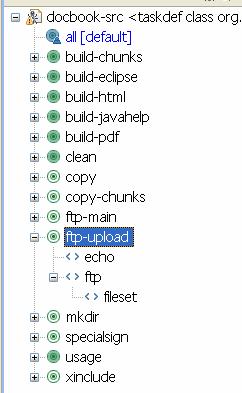
By default Eclipse uses the ant version which ships with Eclipse. Via the | you can configure which version of Ant Eclipse should use.
10. Ant tips
10.1. Converting Paths
Apache Ant allows to convert relative paths to absolute paths. The following example demonstrates that.
<!-- Location of a configuration -->
<property name="my.config" value="../my-config.xml"/>
<makeurl file="${my.config}" property="my.config.url"/>
This property can later be used for example as a parameter.
<param name="highlight.xslthl.config" expression="${my.config.url}"/>
10.2. Regular expressions
Ant can be used to replace text based on regular expressions. The following Ant Target replaces tabs with double spaces.
<target name="regular-expressions">
<!-- Replace tabs with two spaces -->
<replaceregexp flags="gs">
<regexp pattern="(\t)" />
<substitution expression=" " />
<fileset dir="${outputtmp.dir}/">
<include name="**/*" />
</fileset>
</replaceregexp>
</target>
11. Links and Literature
11.2. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting