Eclipse Extension Points. This tutorial describes the definition and usage of the Eclipse Extension Points. The article is written for and Eclipse 4.2 but you can easily adjust it for Eclipse 3.x.
1. Prerequisites for this tutorial
This tutorial assumes that you have basic understanding of development for the Eclipse platform. Please see Eclipse RCP Tutorial or Eclipse Plug-in Tutorial if you need any basic information.
2. Extensions and extension points
Eclipse provides the concept of extensions to contribute functionality to a certain type of API. The type of API is defined by a plug-in via an extension point. Extensions can be contribute by one or more plug-ins.
These extensions and extension points are defined via the plugin.xml file.
This approach is depicted in the following graphic and the terminology is described in the following table.
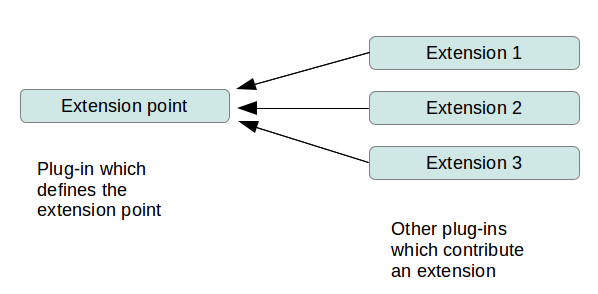
Term | Description |
---|---|
Plug-in defines extension point |
The plug-in defines a contract (API) with the definition of an extension point. This allows other plug-ins to add contributions (extensions) to the extension point. The plug-in which defines the extension point is responsible for evaluating the extensions. Therefore, it typically contains the necessary code to do that. |
A plug-in provides an extension |
This plug-in provides an extension (contribution) based on the contract defined by an existing extension point. Contributions can be code or data. |
3. Creating an extension point
A plug-in which declares an extension point must declare the extension point in its plugin.xml file. You use the Extension Points tab for the definition.
Extension points are described via an XML schema file which is referred to in the plugin.xml file. This XML schema file defines the details and the contract of the extension point. The following code demonstrates the link to the schema file in the plugin.xml file.
<?xml version="1.0" encoding="utf-8"?>
<?eclipse version="3.2"?>
<plugin>
<extension-point id="de.vogella.extensionpoint.greeters"
name="Greeters"
schema="schema/de.vogella.extensionpoint.greeters.exsd" />
</plugin>
The plug-in typically contains code to evaluate the extensions for this extension point.
4. Adding extensions to extension points
The Eclipse IDE provides an editor for the plugin.xml file. It is the same editor which is used for modifying the MANIFEST.MF file.
Select the Extensions tab in this editor to create new extensions. On the Extension Points tab you can define new extension points.
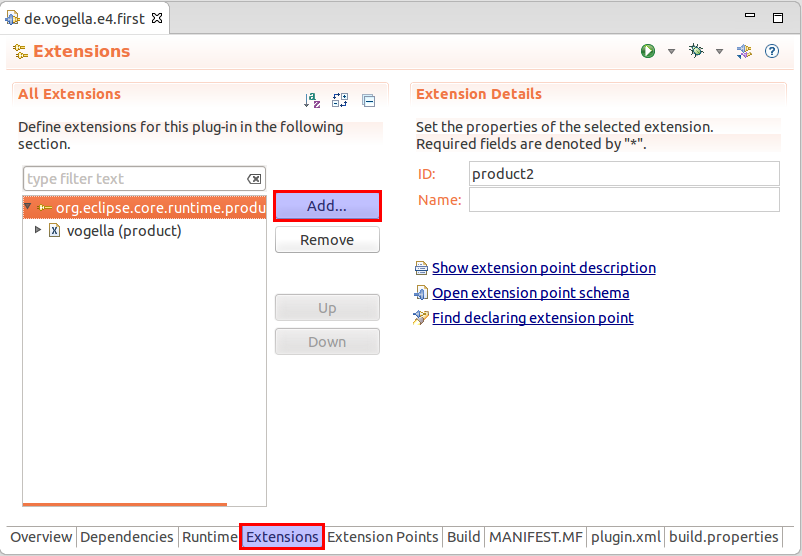
By using the Add… button you can add a new extension. After adding one extension you can right-click on it to add elements or attributes.
5. Accessing extensions
The information about the available extension points and the provided extensions are stored in a class of type IExtensionRegistry
.
The Eclipse platform reads the extension points and provided extensions once the plug-in is in the RESOLVED life cycle status as defined by the OSGi specification. |
In Eclipse applications you can use the dependency injection mechanism to get the IExtensionRegistry
class injected.
@Inject
public void doSomething(IExtensionRegistry registry){
// do something
registry.getConfigurationElementsFor("yourextension");
}
In Eclipse 3.x based plug-ins you query for a certain extension via static methods of the Platform
class.
An example is listed in the following code.
// The following will read all existing extensions
// for the defined ID
Platform.getExtensionRegistry().
getConfigurationElementsFor("yourextension");
You can access extensions of all extension points via the IExtensionRegistery .
|
6. Extension Factories
If you create your class directly via the IConfigurationElement
class of the extension you are limited to classes which have a default constructor.
To avoid this restriction you can use extension factories.
To use a factory implement the interface IExecutableExtensionFactory
in the class attribute of your extension point definition.
The factory receives the configuration elements and can construct the required object.
7. Optional exercise: Create and evaluate an extension point
In this exercise, you create two new plug-ins. The first one contains an extension point. The second plug-in contributes an extension to the new extension point.
7.1. Creating a plug-in for the extension point definition
Create a new plug-in project called com.vogella.extensionpoint.definition based on the settings of the following screenshot.
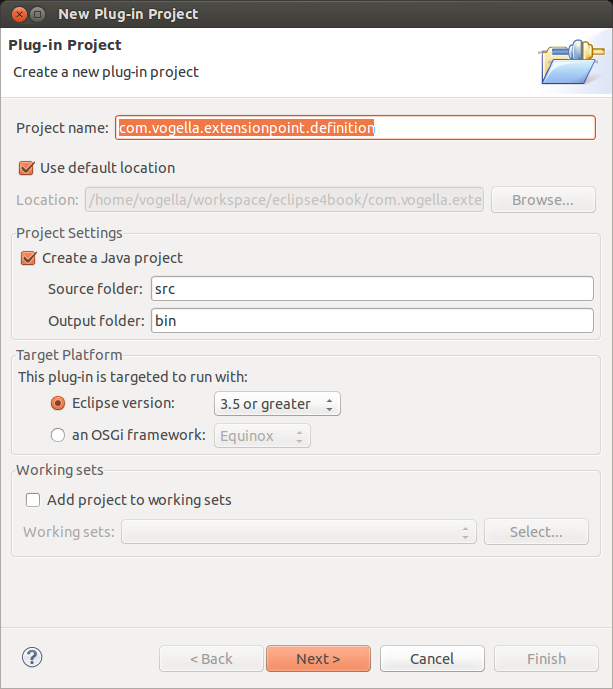
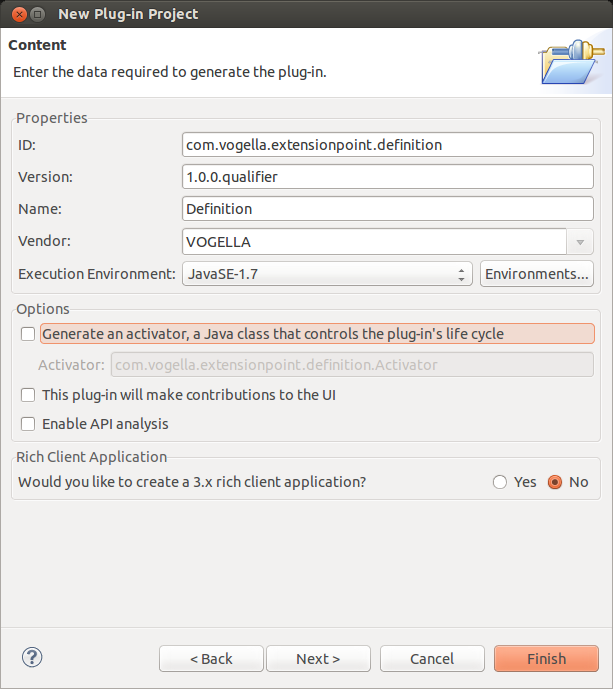
7.2. Create an extension point
Open the MANIFEST.MF file or the plugin.xml file and select the Extension Points tab.

Press the Add… button.
Enter "com.vogella.extensionpoint.definition.greeter" as Extension Point ID and "Greeter" as Extension Point Name in the dialog. The Extension Point Schema field is automatically populated based on your input.
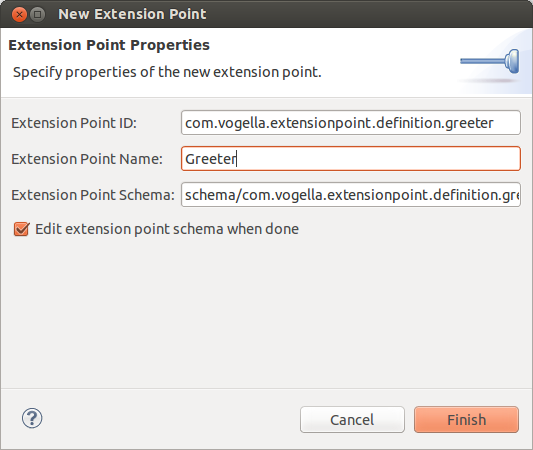
Press the Finish button. The definition of the extension is generated and the schema editor opens. Switch to the Definition tab.

7.3. Add attributes to the extension point.
For that click on the New Element button. Give the new element the name "client".
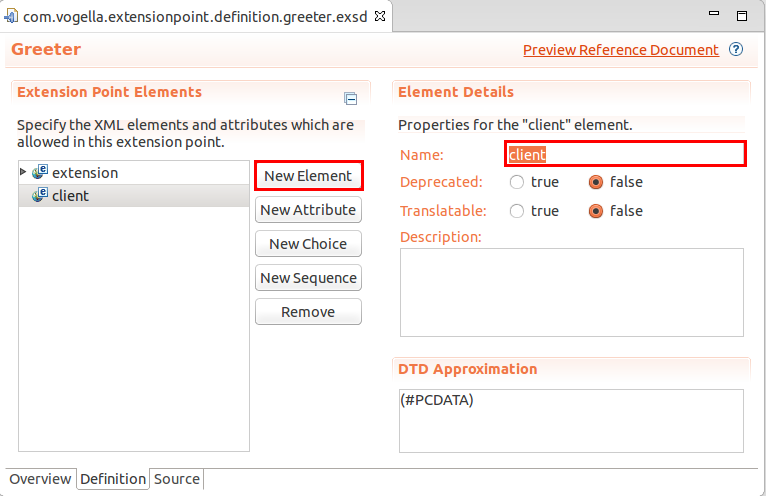
Select the "client" element and press New Attribute.
Give the new element the name "class" and the type "java".
Enter com.vogella.extensionpoint.definition.IGreeter
as interface name
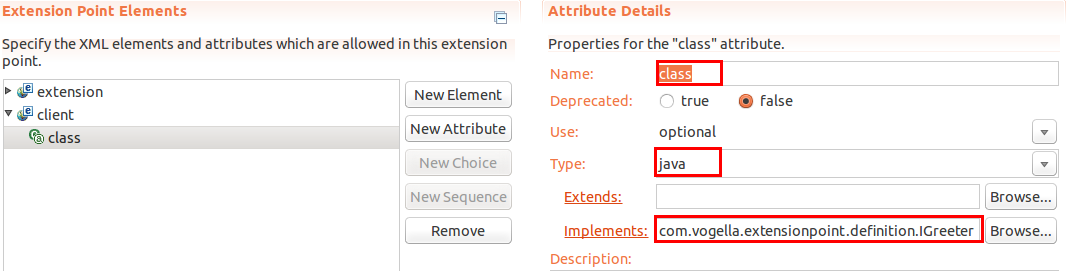
This interface doesn’t exit yet. Press on the hyperlink called Implements to create it based on the following code.
package com.vogella.extensionpoint.definition;
public interface IGreeter {
void greet();
}
Go back to your extension point definition and add a choice to the extension point. For this select the extension entry, right-click on it and select
. This defines how often the extension "client" can be provided by contributing plug-ins. We will set it as unbounded (no restrictions).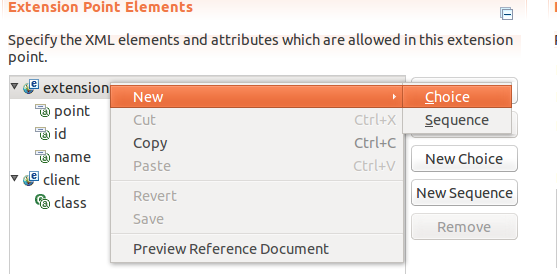
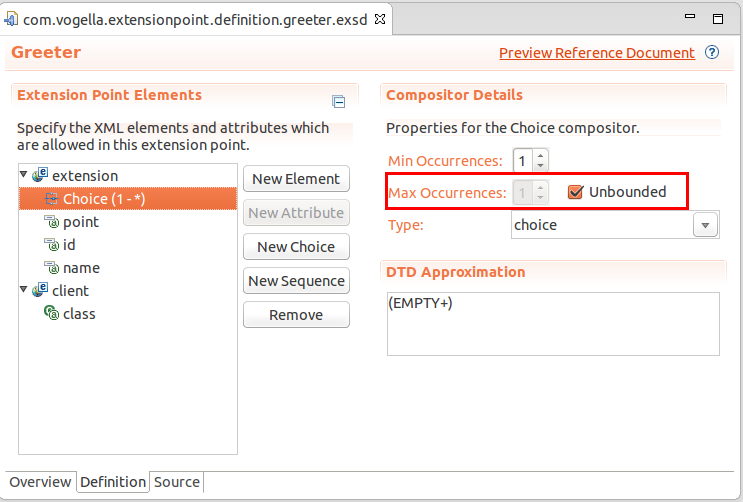

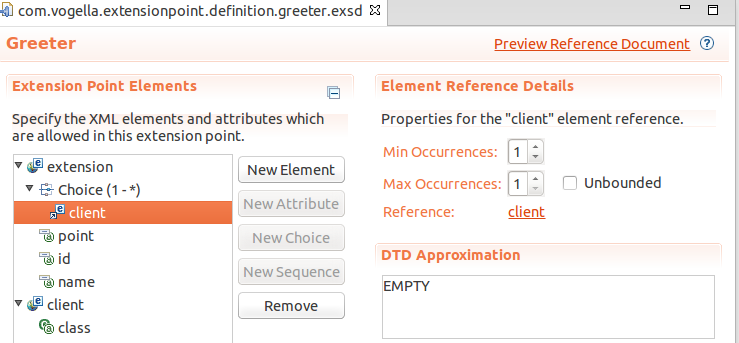
7.4. Export the package
Select the MANIFEST.MF file switch to the Runtime tab and export the package which contains the IGreeter
interface.
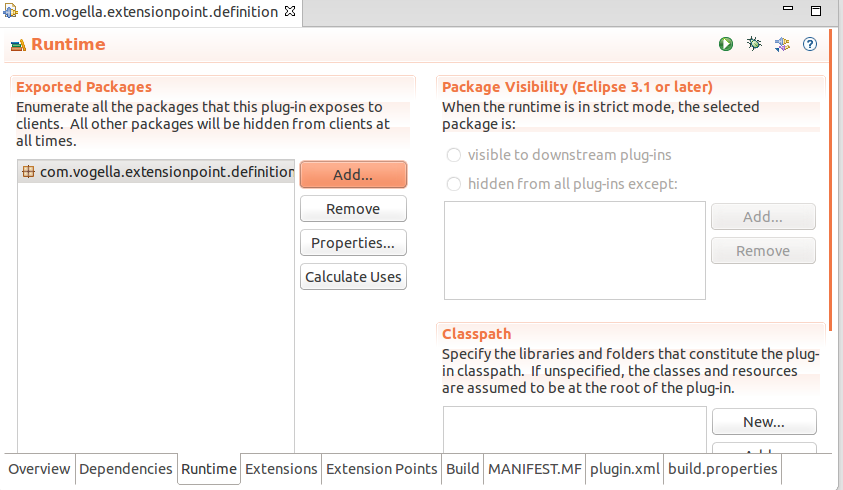
7.5. Add dependencies
Add the following dependencies via the MANIFEST.MF file of your new plug-in:
-
org.eclipse.core.runtime
-
org.eclipse.e4.core.di
7.6. Evaluating the registered extensions
The defining plug-in evaluates the existing extension points. In the following example, you create a handler class to evaluate the existing extensions.
Create the following class.
package com.vogella.extensionpoint.definition;
import org.eclipse.core.runtime.CoreException;
import org.eclipse.core.runtime.IConfigurationElement;
import org.eclipse.core.runtime.IExtensionRegistry;
import org.eclipse.core.runtime.ISafeRunnable;
import org.eclipse.core.runtime.SafeRunner;
import org.eclipse.e4.core.di.annotations.Execute;
public class EvaluateContributionsHandler {
private static final String IGREETER_ID =
"com.vogella.extensionpoint.definition.greeter";
@Execute
public void execute(IExtensionRegistry registry) {
IConfigurationElement[] config =
registry.getConfigurationElementsFor(IGREETER_ID);
try {
for (IConfigurationElement e : config) {
System.out.println("Evaluating extension");
final Object o =
e.createExecutableExtension("class");
if (o instanceof IGreeter) {
executeExtension(o);
}
}
} catch (CoreException ex) {
System.out.println(ex.getMessage());
}
}
private void executeExtension(final Object o) {
ISafeRunnable runnable = new ISafeRunnable() {
@Override
public void handleException(Throwable e) {
System.out.println("Exception in client");
}
@Override
public void run() throws Exception {
((IGreeter) o).greet();
}
};
SafeRunner.run(runnable);
}
}
The code above uses the ISafeRunnable
interface.
This interface protects the plug-in which defines the extension point from malfunction extensions.
If an extension throws an Exception
, it will be caught by ISafeRunnable
and the remaining extensions will still get executed.
Review the .exsd schema file and the reference to this file in the plugin.xml file.
7.7. Create a menu entry and add it to your product
Add a dependency to the com.vogella.extensionpoint.definition
plug-in in the MANIFEST.MF file of your application plug-in.
Afterwards create a new menu entry called Evaluate extensions and define a command and handler for this menu entry.
In the handler point to the EvaluateContributionsHandler
class.
A better approach would be to add the menu entry via a model fragment or a model processor, but I leave that as an additional exercise to the reader. |
7.8. Providing an extension
Create a new simple plug-in called com.vogella.extensionpoint.contribution.
Open the MANIFEST.MF editor of this new plug-in and select the Dependencies tab.
Add the com.vogella.extensionpoint.definition
and org.eclipse.core.runtime
plug-ins as dependencies.
Ensure that the This plug-in is a singleton flag is enabled on the Overview tab of your MANIFEST.MF file.
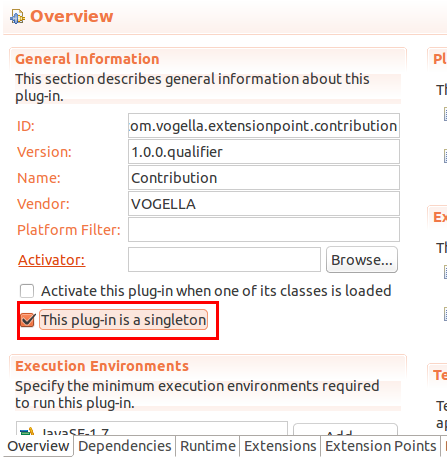
Switch to the Extensions tab and select Add…. Select your custom extension point and press the Finish button.

Add a client element to your extension point via right-click.
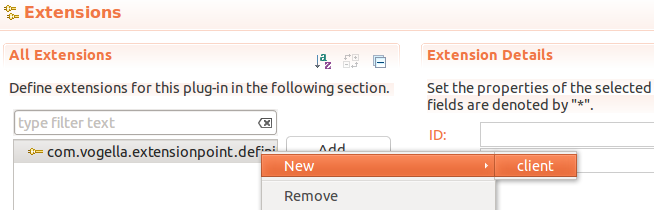
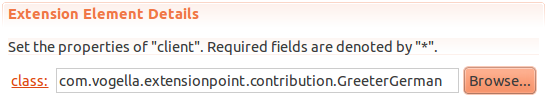
Create the GreeterGerman
class with the following code.
package com.vogella.extensionpoint.contribution;
import com.vogella.extensionpoint.definition.IGreeter;
public class GreeterGerman implements IGreeter {
@Override
public void greet() {
System.out.println("Hallo!");
}
}
7.9. Update product
Add the new plug-ins to your product (via your feature configuration).
-
com.vogella.extensionpoint.contribution
-
com.vogella.extensionpoint.definition
7.10. Validate
Start your application via the product configuration file. This updates the plug-ins included in the run configuration.
In your running application select your new menu entry. The handler class writes the output of your extensions to the Console view of your Eclipse IDE.
8. Links and Literature
8.2. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting