Eclipse Birt. This tutorial describes how to use Eclipse BIRT for reporting on simple Java Objects (POJO’s). The tutorial explains also how to deploy the resulting BIRT report into a webcontainer (Tomcat) and how to use it in an Eclipse RCP application. Eclipse 4.6 (Indigo) is used for this tutorial.
1. Eclipse BIRT
1.1. Overview
Eclipse BIRT allows the creation of reports based on data from different data sources. Data sources define where the data is stored.
BIRT provides for example the following data sources:
-
Databases (via JDBC)
-
Text Files (cvs, XML)
-
WebServices (via WSDL-Files)
-
Scripting Data sources
You use in BIRT Data sets to defines queries on data source. These data sets can be used in a report.
In a Java program it is often convenient to use Java objects as a data source for reports. This article focuses on the usage of Plain Old Java Objects (POJO) as data sources for BIRT reports.
1.2. Example
In this tutorial we will build a report which will show us information about the stock market. We get the information from a Java Object. The data will be displayed in a chart and in a table with detailed information. The result should look like this:
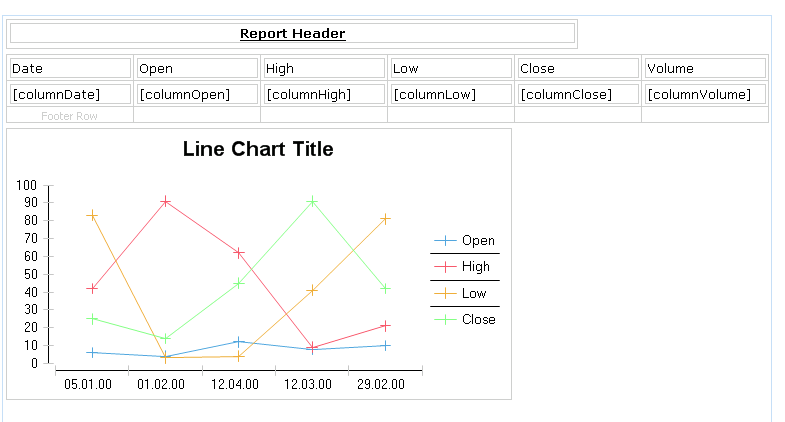
2. Installation
Use the Eclipse Update Manager to install "Business Intelligence, Reporting and Charting" → BIRT Framework.
3. Create Project and REport
Create a new Java Project with the name de.vogella.birt.stocks.
Create a new report stock_report.rptdesign via
.


The new report is displayed in the Report Design perspective. Delete everything in the example report except the report header. The result should look like the following.

4. Java classes
The report will display stock data. To demonstrate BIRT we use a Mock object for providing the data.
Create package de.vogella.birt.stocks.model and then the following class. This class will represent the domain model.
package de.vogella.birt.stocks.model;
import java.util.Date;
/**
* Domain model for stock data
* @author Lars Vogel
*/
public class StockData {
private Date date;
private double open;
private double high;
private double low;
private double close;
private long volume;
public double getClose() {
return close;
}
public void setClose(double close) {
this.close = close;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public double getHigh() {
return high;
}
public void setHigh(double high) {
this.high = high;
}
public double getLow() {
return low;
}
public void setLow(double low) {
this.low = low;
}
public double getOpen() {
return open;
}
public void setOpen(double open) {
this.open = open;
}
public long getVolume() {
return volume;
}
public void setVolume(long volume) {
this.volume = volume;
}
}
Create the package de.vogella.birt.stocks.daomock and then the following class StockDaoMock. This will only mock / fake the data and not really get it from the Internet. As we want to learn BIRT here this should be fine.
package de.vogella.birt.stocks.daomock;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.List;
import de.vogella.birt.stocks.model.StockData;
public class StockDaoMock {
public List<StockData> getStockValues(String company) {
// Ignore the company and always return the data
// A real implementation would of course use the company string
List<StockData> history = new ArrayList<StockData>();
// We fake the values, we will return fake value for 01.01.2009 -
// 31.01.2009
double begin = 2.5;
for (int i = 1; i <= 31; i++) {
Calendar day = Calendar.getInstance();
day.set(Calendar.HOUR, 0);
day.set(Calendar.MINUTE, 0);
day.set(Calendar.SECOND, 0);
day.set(Calendar.MILLISECOND, 0);
day.set(Calendar.YEAR, 2009);
day.set(Calendar.MONTH, 0);
day.set(Calendar.DAY_OF_MONTH, i);
StockData data = new StockData();
data.setOpen(begin);
double close = Math.round(begin + Math.random() * begin * 0.1);
data.setClose(close);
data.setLow(Math.round(Math.min(begin, begin - Math.random() * begin * 0.1)));
data.setHigh(Math.round(Math.max(begin, close) + Math.random() * 2));
data.setVolume(1000 + (int) (Math.random() * 500));
begin = close;
data.setDate(day.getTime());
history.add(data);
}
return history;
}
}
5. Datasource and Dataset
To use Java Objects (POJO’s) as datasource in Eclipse BIRT you have to map the fields of your Java classes to JavaScript. This JavaScript is used in your report and will access the Java Object.
5.1. Create Data Source
The data source connects your data with your report. BIRT provides different types of data sources, we use the "Scripted Data Source". Go back to your stocks_report, use the "Report Design" perspective and select the "Data Explorer" View.
You have to select your report to display the content of the datasource view. |
Create a new datasource, named "srcStocks" in your report.
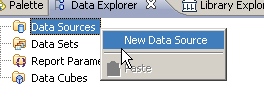

5.2. The Dataset
The dataset defines the mapping for the datasource data and the BIRT data. Create a new dataset named "dataSetSocks".


Press next and define the columns for your report.

5.3. JavaScript
Now we have to write the JavaScript for our dataset. Select the dataset and choose "open" as script. The open script is called before the first access to the dataset. We use this to load our List with the stock objects. To access a Java class you only have to use the following syntax: Packages.myJavaClass where myJavaClass is the full qualified Java class name.
In case you do not see the script please node that the editor for the report has several tab. One of it is labeled "source". |
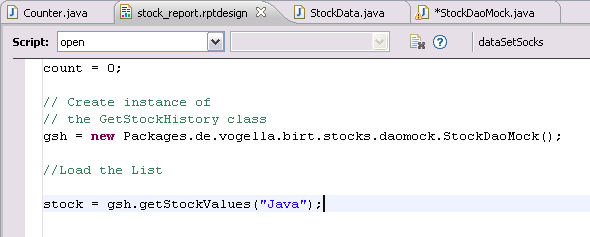
count = 0;
// create instance of
// the GetStockHistory class
gsh = new Packages.de.vogella.birt.stocks.daomock.StockDaoMock();
//Load the List
stock = gsh.getStockValues("Java");
Place the following coding in the fetch script.
if(count < stock.size()){
row["columnDate"] = stock.get(count).getDate();
row["columnOpen"] = stock.get(count).getOpen();
row["columnHigh"] = stock.get(count).getHigh();
row["columnLow"] = stock.get(count).getLow();
row["columnClose"] = stock.get(count).getClose();
row["columnVolume"] = stock.get(count).getVolume();
count++;
return true;
}
return false;
Check if your Script works by doubleclicking on the dataset → Preview Result.

6. Display the data in a table
6.1. Overview
We will now display the data in a table.
6.2. Create a table
Switch from "Data Explorer" to the "Palette". Select the tab "Layout".
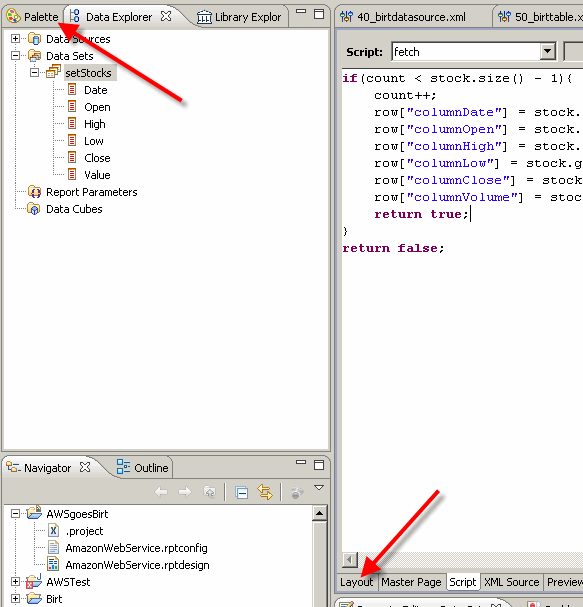
Drag and drop the table element on the report.

Define the following settings for the table.

Change back to the "Data Explorer". And drag and drop the dataset columns into the "Details row" of the table.

The result should look like the following.

Done. You can see a preview of the report if you click on the "Review" Tab. The result should look like the following:
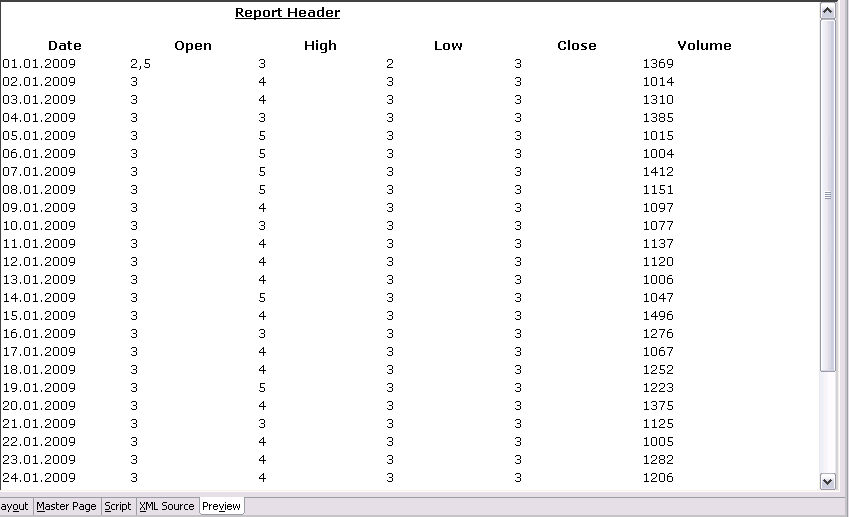
7. Chart
7.1. Create a Chart
Switch back to the Palette, select a chart and drag and drop it on your report. Choose the Line Chart with the standard settings.

Press Next and select your data set.
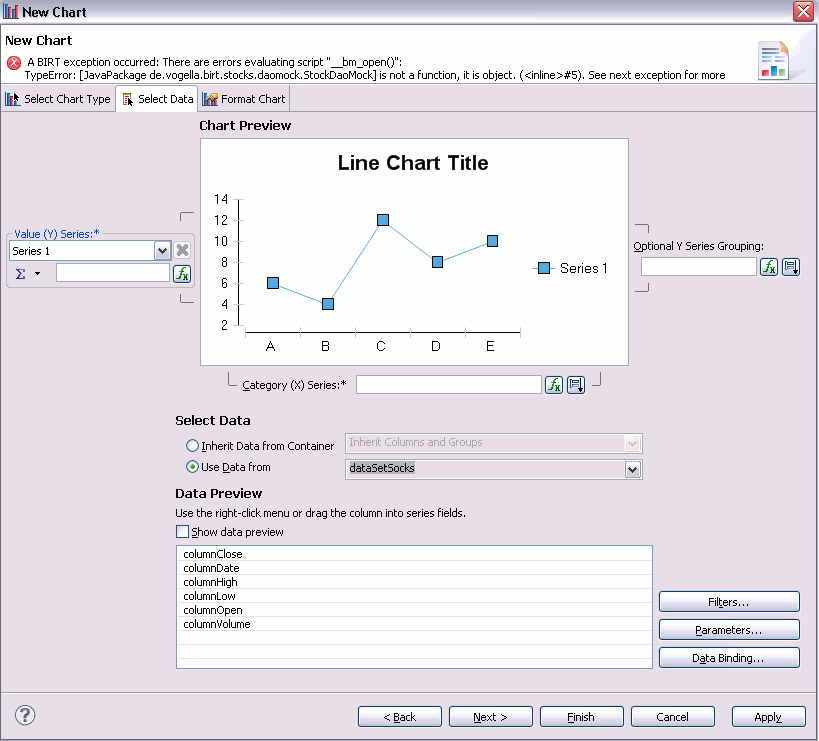
At the next step we have to assign the columns to the axis. We assign the date to the x axis and the open value to the y axis via drag and drop.
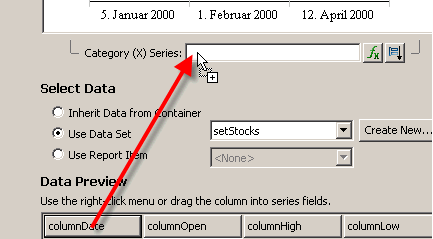
Define 5 series in total. Assign the columns to these series by dragging the column to the Sum sign.

Currently the x axis shows first the newest date. Reverse the x axis by you have to sort the data ascending. Press the highlighted button.

Go to the next tab and give titles to your columns. Hide the last one.
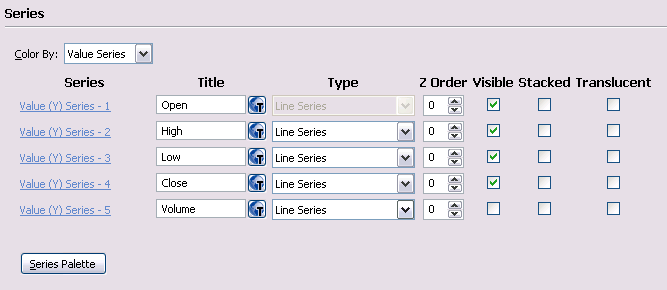
The display of the dates use a long format, we would like to change this. Perform the following and choose "short" as date type of the x axis
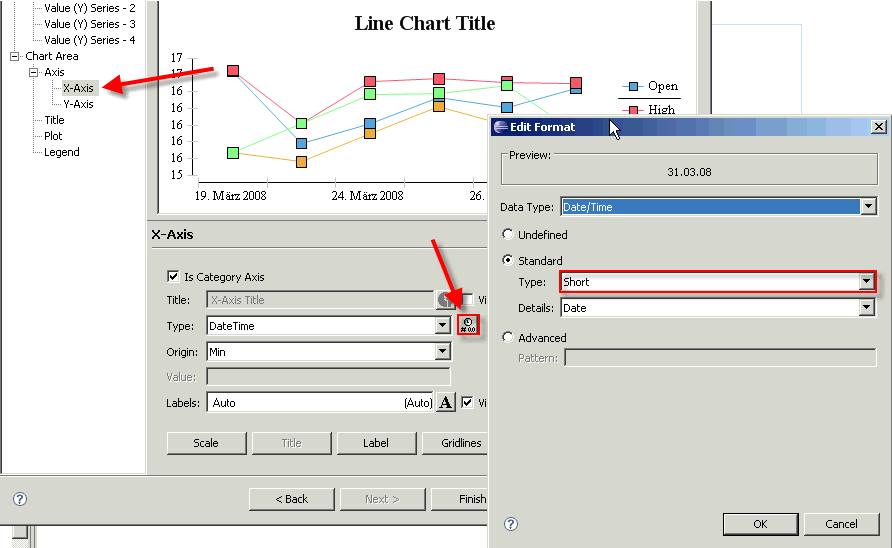
Change the display of the lines via the following.
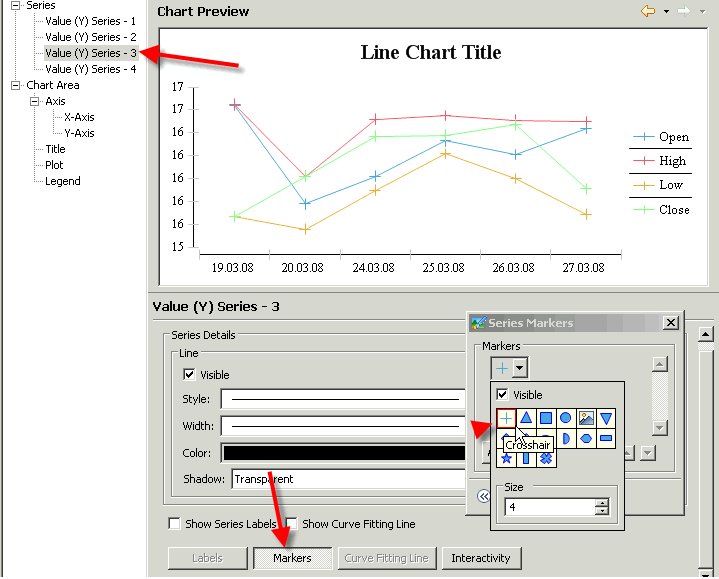
Press finish to include your chart into your report.
8. Deploying in Tomcat
8.1. Overview
The following explains how to use BIRT reports in Tomcat. In general you have to:
-
Install the BIRT webviewer in Tomcat
-
Export your BIRT project into a .jar file
-
Move the .jar file to the birt-install-directory/WEB-INF/lib directory
-
Move the report design file into the root directory of birt in tomcat
-
Restart Tomcat
8.2. Install BIRT in Tomcat
We will use a stand-alone Tomcat 6.0 which we assume is already installed. See Apache Tomcat Tutorial for details.
You need the "Deployment components of BIRT" https://download.eclipse.org/birt/downloads/.
Copy the birt.war of this download into the Tomcat webappsfolder.
Currently you have to install org.eclipse.commons.logging separately into Tomcat. Download this lib from http://commons.apache.org/logging/ and put the jars into the lib folder of Tomcat. |
The Birt example should be available under
http://localhost:8080/birt/
If you see something like this, your Tomcat an your Web Viewer should work correct.
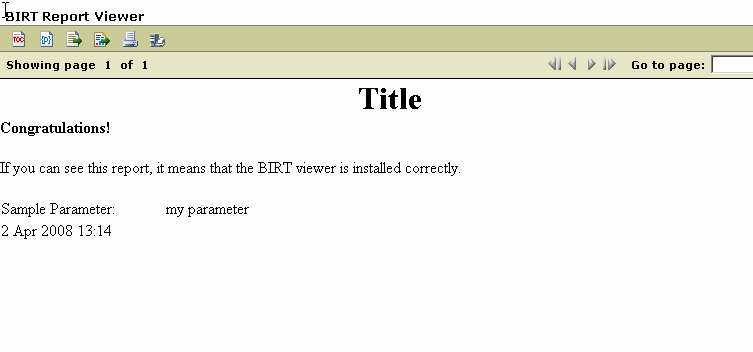
8.3. Install your BIRT reports in Tomcat
To run your own reports you have to copy the .rptdesign file in the root of the birt folder in Tomcat. To make your Java classes available export your project into a jar file.
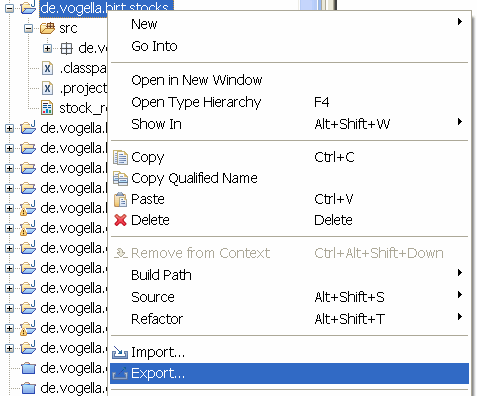

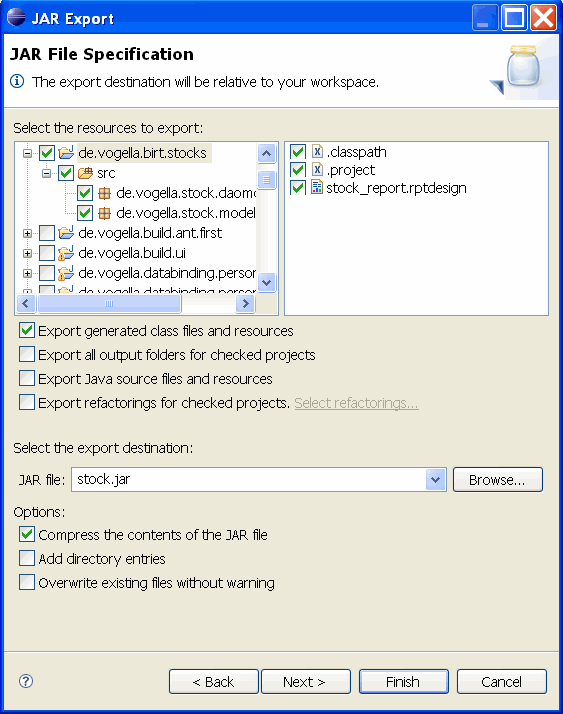
After that the jar file has to be copied to the Tomcat webapps/birt/WEB-INF/lib/ directory. Restart the Tomcat and navigate to your report.
Your report should be found under
http://localhost:8080/birt/frameset?__report=stock_report.rptdesign

If you want to export your report to PDF you also need the library iText from ( http://prdownloads.sourceforge.net/itext/itext-1.3.jar). Copy the iText.jar in "/birt-viewer/WEB-INF/platform/plugins/com.lowagie.itext/lib". Now restart the Tomcat. |
9. Deploying in Eclipse RCP application
9.1. BIRT deploying to an RCP Application
We can use the BIRT viewer in a local RCP Application. It isn’t more than an browser view which shows a HTML Page generated by an integrated Webserver.
The following assumes that you are already familiar with Eclipse RCP development. See Eclipse RCP Tutorial in case you need an introduction.
Convert "de.vogella.birt.stocks" to a plug-in project, via right-click → Configure → "Convert to plug-in project".
Create an new plug-in project "de.vogella.birt.stocks.rcp". Select the template "RCP Application with a view".
Add the following plugins as dependendies to "de.vogella.birt.stocks.rcp".
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-Name: Rcp
Bundle-SymbolicName: de.vogella.birt.stocks.rcp; singleton:=true
Bundle-Version: 1.0.0.qualifier
Bundle-Activator: de.vogella.birt.stocks.rcp.Activator
Require-Bundle: org.eclipse.ui,
org.eclipse.core.runtime,
org.eclipse.birt.report.viewer,
org.eclipse.birt.report.engine.emitter.html,
de.vogella.birt.stocks,
org.eclipse.birt,
org.eclipse.birt.chart.ui,
org.eclipse.birt.chart.device.extension,
org.eclipse.birt.chart.device.pdf,
org.eclipse.birt.chart.device.svg,
org.eclipse.birt.chart.device.swt,
org.eclipse.birt.chart.engine.extension,
org.eclipse.birt.chart.reportitem,
org.eclipse.birt.chart.reportitem.ui,
org.eclipse.birt.chart.ui.extension,
org.eclipse.birt.core.script.function,
org.eclipse.birt.report.engine.script.javascript
Bundle-ActivationPolicy: lazy
Bundle-RequiredExecutionEnvironment: JavaSE-1.6
Copy your report to "stock_report_rcp.rptdesign" into this new project. Open this report and change the "open" JavaScript to the following.
count = 0;
/*
* load and init data reader
* import Platform from org.eclipse.core.runtime
*/
importPackage(Packages.org.eclipse.core.runtime);
/* load bundle with POJOs and data loading class */
myBundle = Platform.getBundle("de.vogella.birt.stocks");
/* load data reader class */
readerClass = myBundle.loadClass("de.vogella.birt.stocks.daomock.StockDaoMock");
/* create new instance of DataReader */
readerInstance = readerClass.newInstance();
/* read data */
stock = readerInstance.getStockValues("Java");
Use this code as View.java.
package de.vogella.birt.stocks.rcp;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import org.eclipse.birt.report.viewer.utilities.WebViewer;
import org.eclipse.core.runtime.FileLocator;
import org.eclipse.core.runtime.Path;
import org.eclipse.core.runtime.Platform;
import org.eclipse.swt.SWT;
import org.eclipse.swt.browser.Browser;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.ui.part.ViewPart;
import org.osgi.framework.Bundle;
public class View extends ViewPart {
public static final String ID = "de.vogella.birt.stocks.rcp.view";
public void createPartControl(Composite parent) {
String path = "";
try {
Bundle bundle = Platform.getBundle("de.vogella.birt.stocks.rcp");
URL url = FileLocator.find(bundle, new Path(
"stock_report_rcp.rptdesign"), null);
path = FileLocator.toFileURL(url).getPath();
} catch (MalformedURLException me) {
System.out.println("Fehler bei URL " + me.getStackTrace());
} catch (IOException e) {
e.printStackTrace();
}
Browser browser = new Browser(parent, SWT.NONE);
// use the filename of your report
WebViewer.display(path, WebViewer.HTML, browser, "frameset");
}
/**
* Passing the focus request to the viewer's control.
*/
public void setFocus() {
}
}
10. Links and Literature
10.1. Eclipse BIRT resources
If you need more assistance we offer Online Training and Onsite training as well as consulting