Python, Pydev and Eclipse. This article describes how to write and debug Python programs with Eclipse This article is based on Eclipse 4.3, Python 3.3.1 and PyDev version 2.7.3.
1. Overview
1.1. What is Python
Python is an interpreted programming language and claims to be a very effective programming language. Python was develop by Guido van Rossum.
The name Python is based on the TV show called Monty Python’s Flying Circus. During execution the Python source code is translated into bytecode which is then interpreted by the Python interpreter. Python source code can also run on the Java Virtual Machine, in this case you are using Jython.
Key features of Python are:
-
high-level data types, as for example extensible lists
-
statement grouping is done by indentation instead of brackets
-
variable or argument declaration is not necessary
-
supports for object-orientated, procedural and functional programming style
1.2. Block concept in Python via indentation
Python identify blocks of code by indentation. If you have an if statement and the next line is indented then it means that this indented block belongs to the if. The Python interpreter supports either spaces or tabs, e.g. you cannot mix both. The most "pythonic" way is to use 4 spaces per indentation level.
1.3. About this tutorial
This tutorial will first explain how to install Python and the Python plugins for Eclipse. It will then create a small Python project to show the usage of the plugin. Afterwards the general constructs of Python are explained.
2. Installation
2.1. Python
Download Python from http://www.python.org. Download the version 3.3.1 or higher of Python. If you are using Windows you can use the native installer for Python.
2.2. Eclipse Python plugin
The following assume that you have already Eclipse installed. For an installation description of Eclipse please see Eclipse IDE for Java.
For Python development under Eclipse you can use the PyDev Plugin which is an open-source project. Install PyDev via the Eclipse update manager via the following update site: http://pydev.org/updates.
2.3. Configuration of Eclipse
You also have to maintain in Eclipse the location of your Python installation. Open in the
menu.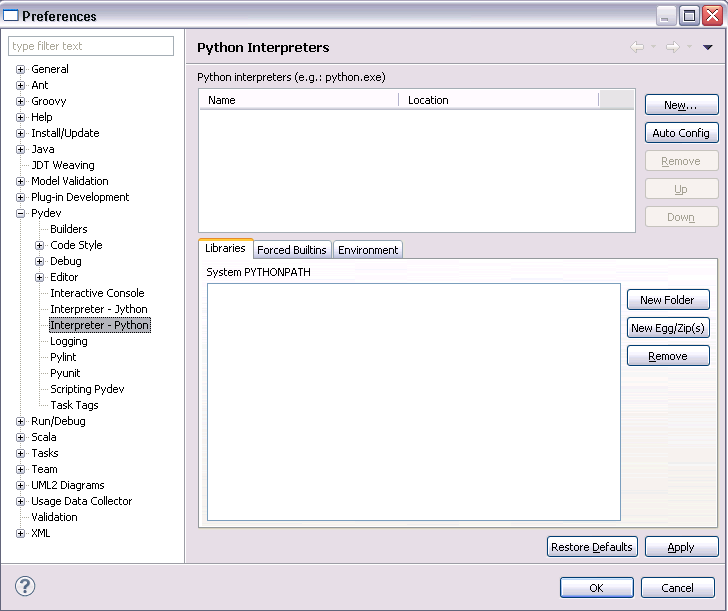
Press the
New
button
and enter the path to
python.exe
in your
Python installation directory. For Linux and macOS users
this is
normally /usr/bin/python.
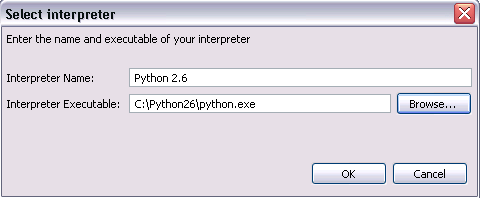
Note that on MAC OS X the python libraries are not installed by default. Please use Google to find out, how to install them on a Mac OS X system, the author of this text only uses Linux. |
The result should look like the following.
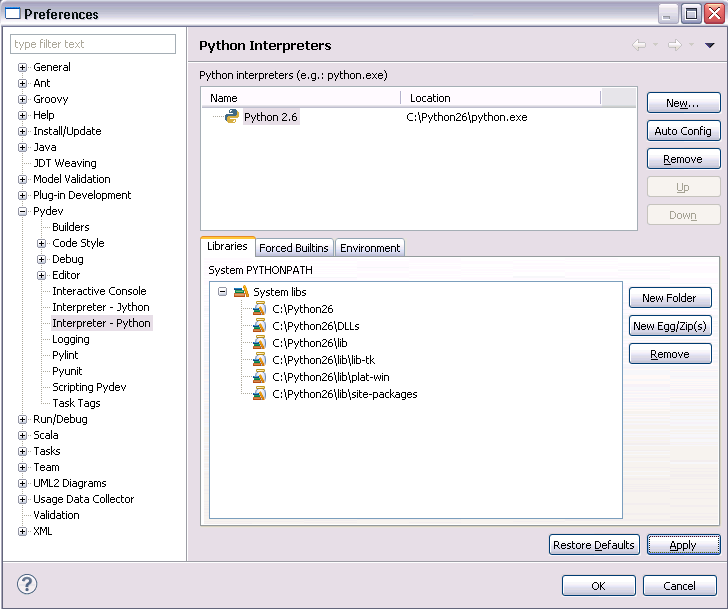
3. Your first Python program in Eclipse
Select
. Select Pydev → Pydev Project.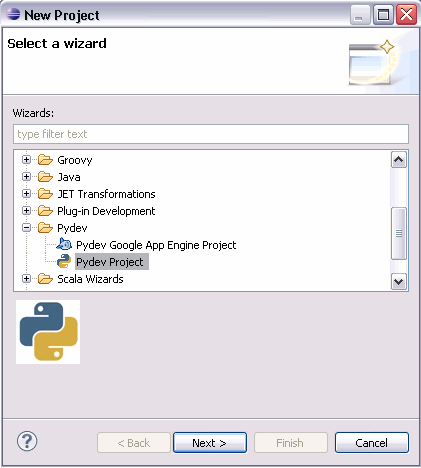
Create a new project with the name "de.vogella.python.first". Select Python version 2.6 and your interpreter.
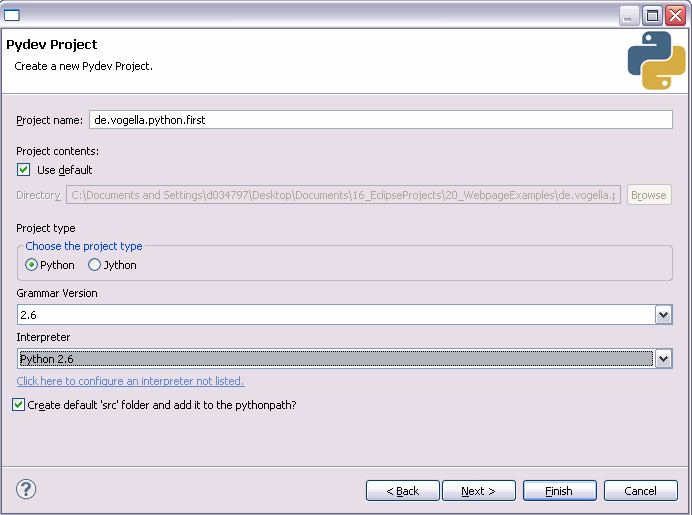
Press finish.
Select
. Select the PyDev perspective.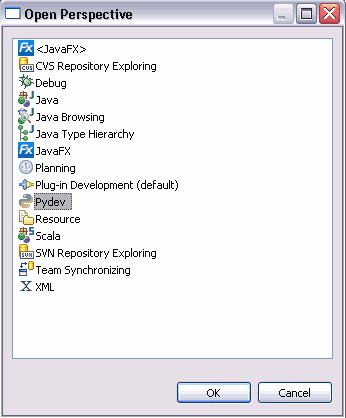
Select the "src" folder of your project, right-click it and select New → PyDev Modul. Create a module "FirstModule".
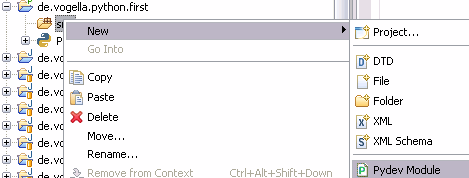
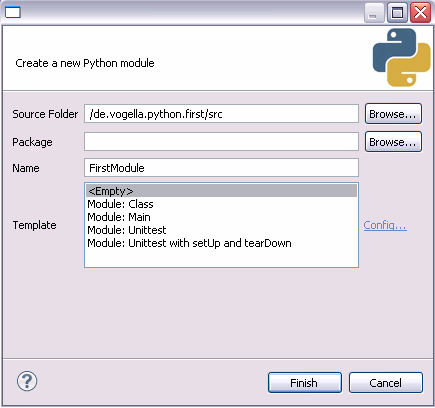
Create the following source code.
'''
Created on 18.06.2009
@author: Lars Vogel
'''
def add(a,b):
return a+b
def addFixedValue(a):
y = 5
return y +a
print add(1,2)
print addFixedValue(1)
Right-click your model and select Run As → Python run.
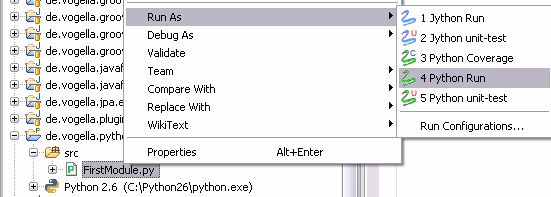
Congratulations! You created your first (little) Python modul and ran it!
4. Debugging
Just right-click in the source code and add a breakpoint.
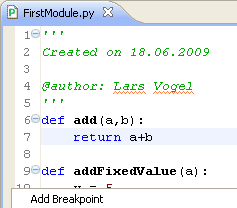
Then select Debug as → Python Run
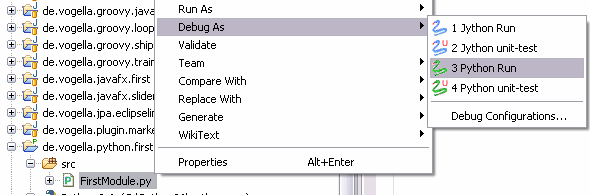
You can now inspect and modify the variables in the variables view.
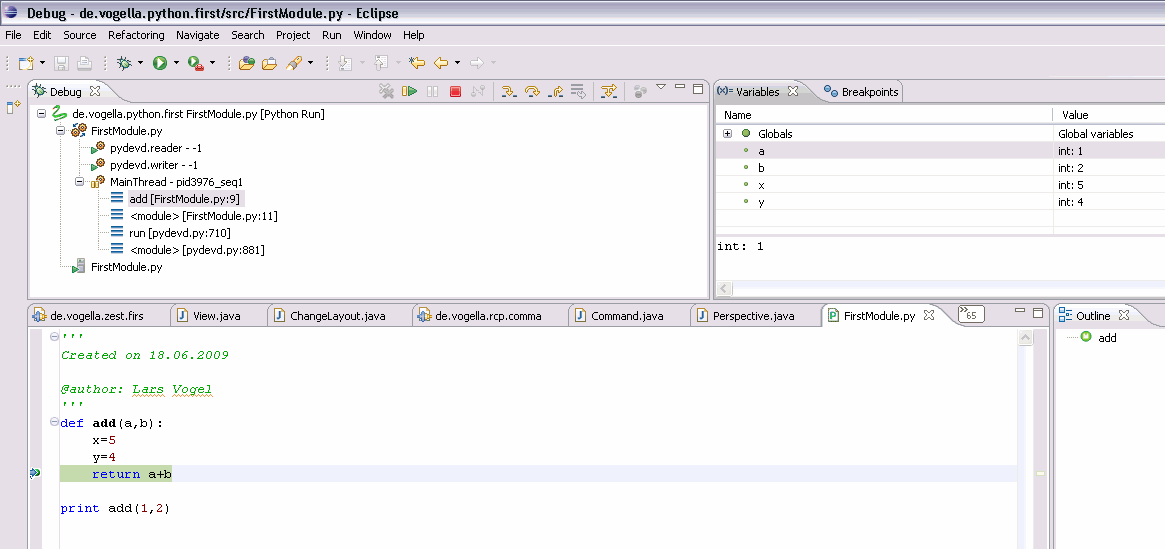
Via the debug buttons (or shortcuts F5, F6, F7, F8) you can move in your program.
You can use F5 / F6, F7 and F8 to step through your coding.
Command | Description |
---|---|
F5 |
Goes to the next step in your program. If the next step is a method / function this command will jump into the associated code. |
F6 |
F6 will step over the call, e.g. it will call a method / function without entering the associated code. |
F7 |
F7 will go to the caller of the method/ function. So this will leave the current code and go to the calling code. |
F8 |
Use F8 to go to the next breakpoint. If no further breakpoint is encountered then the program will normally run. |
You can of course use the ui to debug. The following displays the key bindings for the debug buttons.
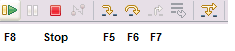
5. Programming in Python
5.2. Variables
Python provides dynamic typing of its variables, e.g. you do not have to define a type of the variable Python will take care of this for you.
# This is a text
s= "Lars"
# This is an integer
x = 1
y=4
z=x+y
5.4. Methods / Functions in Python
Python allows to define methods via the keyword def. As the language is interpreted the methods need to be defined before using it.
def add(a,b):
return a+b
print add(1,2)
5.5. Loops and if clauses
The following demonstrates a loop the usage of an if-clause.
i = 1
for i in range(1, 10):
if i <= 5 :
print 'Smaller or equal than 5.\n',
else:
print 'Larger than 5.\n',
5.6. String manipulation
Python allows the following String operations.
Operations | Description |
---|---|
len(s) |
Returns the length of string s |
s[i] |
Gets the element on position i in String s, position start with zero |
s[-i] |
Get the i-tes Sign of the string from behind the string, e.g. -1 returns the last element in the string |
"abcdefg"[0:4] |
Gets the first 4 elements (abcd) |
"abcdefg"[4:] |
Gets the elements after the first 4 elements (abcd) |
|
Concatenates the int varibles a, b,c, e.g. if a=1, b=2, c=3 then the result is 123. |
s.lower() |
Result will be s in lower cases |
s.upper() |
Result will be s in upper cases |
s.startswith(t) |
True, if s startsWith t |
s.rstrip() |
Removes the end of line sign from the string |
For example:
s = "abcdefg"
assert (s[0:4]=="abcd")
assert ( s[4:]=="efg")
assert ("abcdefg"[4:0]=="")
assert ("abcdefg"[0:2]=="ab")
5.7. Concatenate strings and numbers
Python does not allow to concatenate a string directly with a number. It requires you to turn the number first into a string with the str() function.
If you do not use str() you will get "TypeError: cannot concatenate 'str' and 'int' objects".
For example:
print 'this is a text plus a number ' + str(10)
5.8. Lists
Python has good support for lists. See the following example how to create a list, how to access individual elements or sublists and how to add elements to a list.
'''
Created on 14.09.2010
@author: Lars Vogel
'''
mylist = ["Linux", "Mac OS" , "Windows"]
# Print the first list element
print(mylist[0])
# Print the last element
# Negativ values starts the list from the end
print(mylist[-1])
# Sublist - first and second element
print(mylist[0:2])
# Add elements to the list
mylist.append("Android")
# Print the content of the list
for element in mylist:
print(element)
If you want to remove the duplicates from a list you can use:
mylist = ["Linux", "Linux" , "Windows"]
# remove duplicates from the list
mylist = list(set(mylist))
5.9. Processing files in Python
The following example is contained in the project "de.vogella.python.files". The following reads a file, strips out the end of line sign and prints each line to the console.
'''
Created on 07.10.2009
@author: Lars Vogel
'''
f = open('c:\\temp\\wave1_new.csv', 'r')
print f
for line in f:
print line.rstrip()
f.close()
The following reads the same file but write the output to another file.
'''
@author: Lars Vogel
'''
f = open('c:\\temp\\wave1_new.csv', 'r')
output = open('c:\\temp\\sql_script.text', 'w')
for line in f:
output.write(line.rstrip() + '\n')
f.close()
5.10. Splitting strings and comparing lists.
The following example is contained in the project "de.vogella.python.files". It reads to files which contain one long comma separated string. This string is splitted into lists and the lists are compared.
f1 = open('c:\\temp\\launchconfig1.txt', 'r')
s= ""
for line in f1:
s+=line
f1.close()
f2 = open('c:\\temp\\launchconfig2.txt', 'r')
s2= ""
for line in f2:
s2+=line
f2.close()
list1 = s.split(",")
list2 = s2.split(",");
print(len(list1))
print(len(list2))
difference = list(set(list1).difference(set(list2)))
print (difference)
5.11. Writing Python Scripts in Unicode
If you read special non ASCII sign, e.g. ö, ä. ü or ß, you have to tell Python which character set to use. Include the following in the first or second line of your script.
# -*- coding: UTF-8 -*-
5.12. Classes in Python
The following is a defined class in Python. Python uses the naming convension _name_ for internal functions. Python allows operator overloading, e.g. you can define what the operator + will do for a specific class.
_init_ | Constructor of the class |
---|---|
_str_ |
The method which is called if print is applied to this object |
_add_ |
+ Operator |
_mul_ |
* Operator |
The empty object (null) is called _None_ in Python.
class Point:
def __init__(self, x=0, y=0):
self.x = x
self.y = y
def __str__(self):
return "x-value" + str(self.x) + " y-value" + str(self.y)
def __add__(self,other):
p = Point()
p.x = self.x+other.x
p.y = self.y+other.y
return p
p1 = Point(3,4)
p2 = Point(2,3)
print p1
print p1.y
print (p1+p2)
6. Google App Engine
Google offers free hosting of small Python based web application. Please see Google App Engine development with Python.
7. Links and Literature
7.1. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting