Lars Vogel, (c) 2013, 2024 vogella GmbH Version 1.1, 03.06.2021
This tutorial describes the usage of a Jetty Server standalone and embedded in a Java application.
1. What is Jetty?
Jetty is a web server which can be easily embedded into Java applications. It has the following core components:
-
Http Server
-
Servlet Container
-
HTTP Client
2. Using Jetty as standalone server
2.1. Installation of a standalone Jetty
Download Jetty from https://www.eclipse.org/jetty/download.html and extract the zip file somewhere to your filesystem.
This update site contains also a Eclipse p2 update site, in case you want to use Jetty in an OSGi environment.
2.2. Start Jetty standalone from the JAR file
To start Jetty, switch on the command line to the installation directory and issue the following command.
java -jar start.jar
This starts Jetty on
http://localhost:8080/
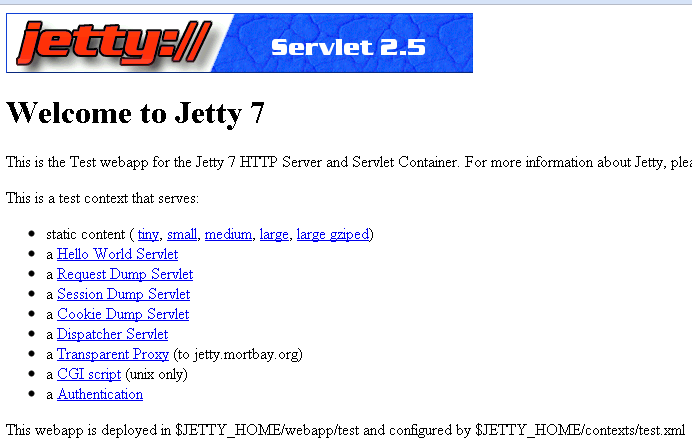
To stop Jetty press Ctrl+C.
To start Jetty as Windows service you can use Apache Procrun. |
3. Embedded Jetty Server in Java with Maven
Create a new Maven project named com.vogella.maven.jetty
.
Update your Java version to at least Java 11 and add the dependencies to Jetty to your pom file.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.vogella</groupId>
<artifactId>com.vogella.maven.jetty</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>com.vogella.maven.jetty</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-server</artifactId>
<version>11.0.3</version>
</dependency>
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-servlet</artifactId>
<version>11.0.3</version>
</dependency>
</dependencies>
</project>
You find the latest version of Jetty Maven on https://search.maven.org/artifact/org.eclipse.jetty/jetty-server |
Create the following classes.
package com.vogella.maven.jetty;
import java.io.IOException;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
public class ExampleServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("application/json");
response.setStatus(HttpServletResponse.SC_OK);
response.getWriter().println("{ \"status\": \"ok\"}");
}
}
The following is a small client:
package com.vogella.maven.jetty;
import java.io.IOException;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.net.http.HttpResponse.BodyHandlers;
public class HttpClientExample {
public static String callServer() {
HttpClient client = HttpClient.newBuilder().build();
HttpRequest request = HttpRequest.newBuilder().GET().uri(URI.create("http://localhost:8082")).build();
try {
HttpResponse<String> response = client.send(request, BodyHandlers.ofString());
if (response.statusCode() == 200) {
return response.body();
} else
return String.valueOf(response.statusCode());
} catch (IOException | InterruptedException e) {
throw new RuntimeException(e.getMessage());
}
}
}
And this code starts a server, register the servlet and uses the client for demo purposes.
package com.vogella.maven.jetty;
import org.eclipse.jetty.server.Connector;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.server.ServerConnector;
import org.eclipse.jetty.servlet.ServletHandler;
public class StartJettyWebserver {
public static void main(String[] args) throws Exception {
var server = new Server(8082);
var handler = new ServletHandler();
server.setHandler(handler);
handler.addServletWithMapping(ExampleServlet.class, "/*");
server.start();
server.join();
}
}
4. Embedd Jetty in an Eclipse RCP application
To use Jetty in an RCP application, add the libraries to your target platform.
<location includeDependencyScope="compile" includeSource="true" missingManifest="generate" type="Maven">
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-server</artifactId>
<version>11.0.3</version>
</location>
<location includeDependencyScope="compile" includeSource="true" missingManifest="generate" type="Maven">
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-servlet</artifactId>
<version>11.0.3</version>
</location>
Add the necessary plug-in to a new feature.
<?xml version="1.0" encoding="UTF-8"?>
<feature
id="rcp.feature.jetty"
label="Jetty"
version="1.0.0.qualifier">
<plugin
id="org.eclipse.jetty.servlet"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.servlet-api"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.http"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.server"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.util"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.io"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="slf4j.api"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
<plugin
id="org.eclipse.jetty.security"
download-size="0"
install-size="0"
version="0.0.0"
unpack="false"/>
</feature>
Add this new feature your product.
You also need to add the Jetty libs to your manifest as dependencies.
// more entries in the manifest
Require-Bundle: org.eclipse.swt;bundle-version="3.114.100",
org.eclipse.jetty.server;bundle-version="11.0.3",
org.eclipse.jetty.http;bundle-version="11.0.3",
org.eclipse.jetty.servlet;bundle-version="11.0.3",
org.eclipse.jetty.util;bundle-version="11.0.3",
org.eclipse.jetty.servlet-api;bundle-version="5.0.2"
Now you can use it in code similar to the Maven example.
5. Jetty resources
If you need more assistance we offer Online Training and Onsite training as well as consulting