This article describes how to use Java libraries for Eclipse plug-in and OSGi development.
1. Using Java libraries in Eclipse plug-ins and OSGi bundles
Java libraries are delivered as JAR files. Eclipse plug-ins and OSGi based applications require additional meta-data in the META-INF/MANIFEST.MF file of libraries which they consume. In case a Java library does not provide the additional meta-data, you can convert the JAR file to an plug-in:
-
Use the Eclipse Maven M2e extension to add Maven libraries to your target platform
-
Manually convert the JAR file to a plug-in via an Eclipse wizard
-
Use Gradle plug-ins to converts JARs
-
Use Maven plug-ins to convert JARs
The recommended way is to use the Eclipse Maven (M2E) extension to add such libraries to your target platform. M2E allows to convert non OSGi Java libraries automatically if they are part of the target platform.
A target platform containing m2e extensions is supported by the Maven Tycho build as of version 2.2.
1.1. Adding a dependency to your target file
The following is an example how to add a library as dependency, while excluding a certain transitive library.
<?xml version="1.0" encoding="UTF-8" standalone="no"?>
<?pde version="3.8"?>
<target name="test" sequenceNumber="170">
<location includeDependencyDepth="infinite" includeDependencyScopes="compile" includeSource="true" label="EclipseLink" missingManifest="generate" type="Maven">
<feature id="eclipselink" label="EclipseLink" version="1.0.0.qualifier" />
<dependencies>
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>org.eclipse.persistence.jpa</artifactId>
<version>4.0.0</version>
<type>jar</type>
</dependency>
<!-- Fuer Lazy Loading
Achtung: Build schlaegt fehl wenn einkommentiert:
Missing requirement: org.eclipse.angus.mail 1.0.0 requires 'java.package; sun.security.util 0.0.0' but it could not be found
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>eclipselink</artifactId>
<version>4.0.0</version>
<type>jar</type>
</dependency>
-->
</dependencies>
<exclude>org.apache.ant:ant:1.10.12</exclude>
<!-- PS: Fuer org.eclipse.persistence:eclipselink wird folgende Abhängigkeit benötigt -->
<exclude>jakarta.transaction:jakarta.transaction-api:2.0.1</exclude>
</location>
</locations>
</target>
2. Installation of the M2E - PDE integration
To use the Maven library integration in your target platform you have to install the corresponding tooling into your IDE.
You can use the following update site to install it via
.https://download.eclipse.org/technology/m2e/releases/latest/
Install m2e PDE Integration, this will install the basic m2e functionality.
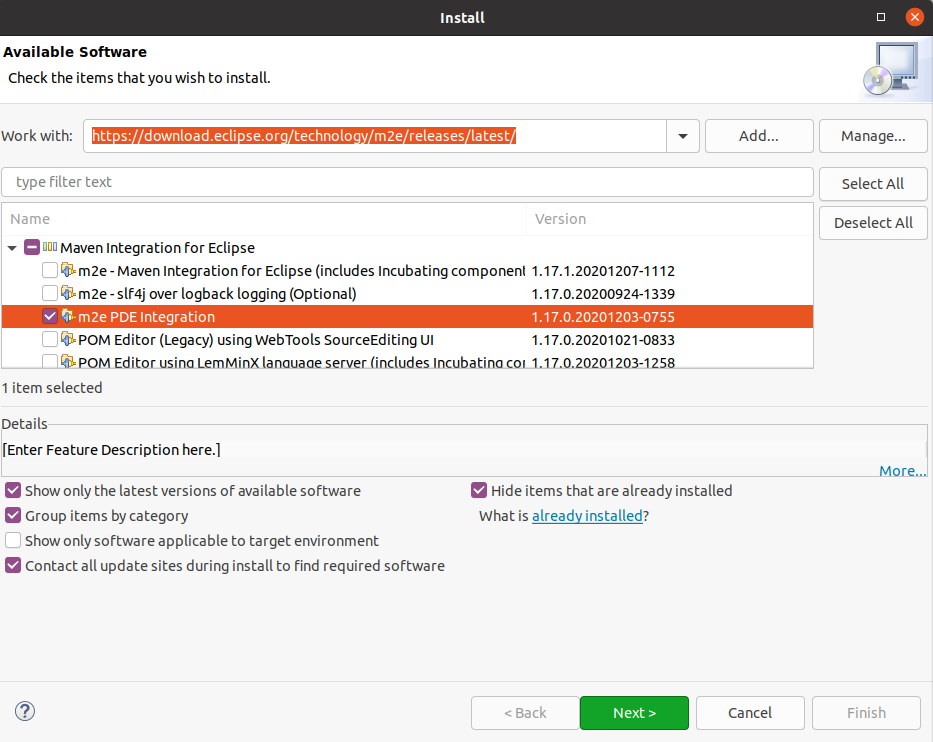
Restart your IDE after the installation.
3. Prerequisites
The following assumes that you already created a target platform for development.
Perform Setting up a target platform if you have not yet done so.
4. Exercise: Make a Maven library available for plug-in development
In this exercise, you add a standard library to your target platform.
4.1. Add GSON library from Maven to your target platform
Open your target platform definition file and press the Add… button on the first tab.
Select Maven in the following dialog.
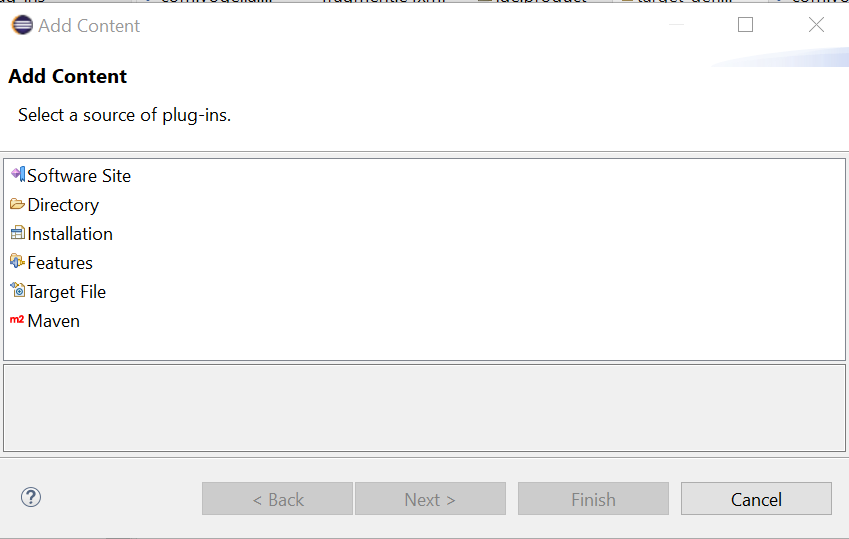
The GSON Maven coordinates we use are listed below.
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.9.0</version>
</dependency>
If you copy a Maven dependency into the clipboard before pressing the add button, the information is used to populate the wizard. |
Select the entries similar to the following.
Also select the option to create a feature.
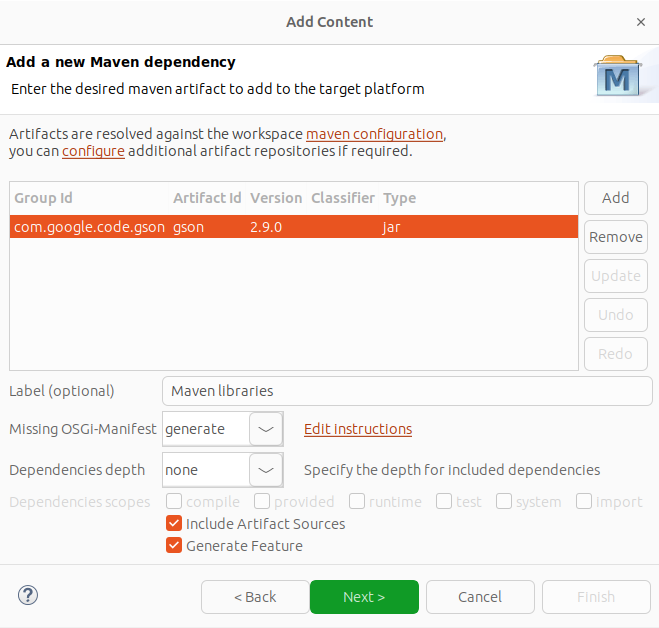
The Dependencies depth and Dependencies scope defines if dependent artifacts are included or only the master artifact itself. If you selected the entries similar to the screenshot, you would download dependencies of the library, the current GSON library has not dependencies. |
Define the feature attribute on the next wizard page.
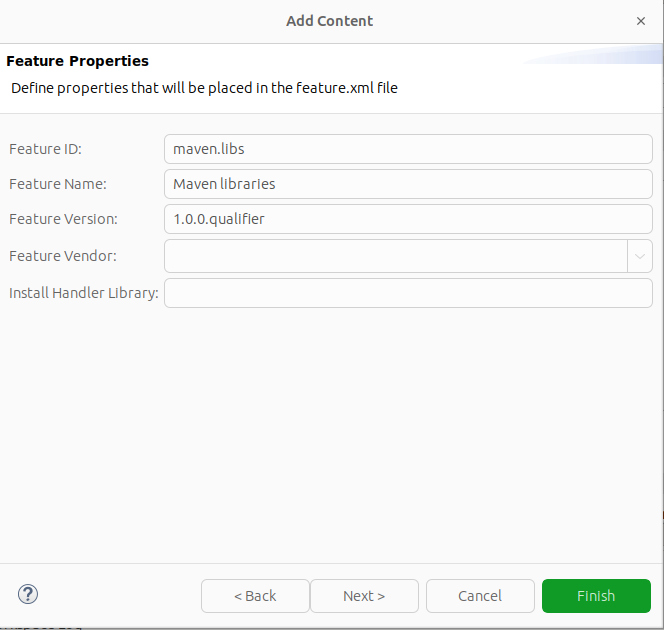
Press Finish. If you press next you could add additional plug-ins from your target platform to the generated feature.
You can now use the library for your plug-in development, even if the original artifact did not contain the OSGi meta-data.
4.2. Validate availability of the library
To test if the library is available for you to develop, open one manifest file for an existing plug-in and switch to the dependency tab. Press Add… and ensure that you can add the library as dependency.
Version in the screenshot has been overdrawn, as this might change over time.
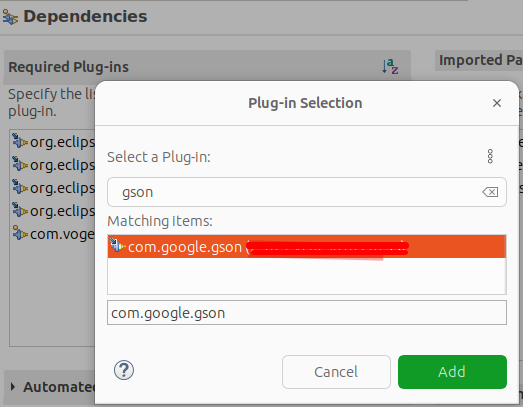
Sometimes you have to restart the IDE to make the update of the target platform available for the MANIFEST.MF editor. |
4.3. Add the feature to your product
Add the generated feature to your product and start your application.
4.4. How to exclude a certain dependency if you include the dependencies of a library
This is added here for further information but it not required to exclude something for this exercise. |
You can exclude dependencies of libraries. At the time of this writing, this does not follow the Maven syntax, see https://github.com/eclipse-m2e/m2e-core/issues/286.
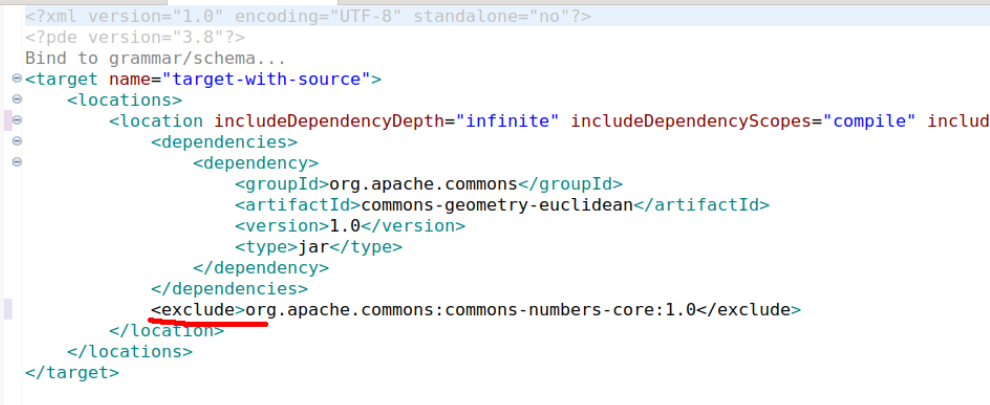
5. Links and Literature
5.1. vogella Java example code
endifdef::printrun[]
If you need more assistance we offer Online Training and Onsite training as well as consulting