Eclipse Advanced Commands. This article describes use cases for Eclipse commands which go beyond the simple one for adding commands to menus, toolbars, etc. This article is based on Eclipse Indigo (3.7).
This tutorial describes the Eclipse 3.x API which is used for Eclipse IDE plug-in development. For Eclipse RCP you should use the new Eclipse 4 API which is described in the Eclipse RCP tutorial. |
1. Eclipse Commands
For an introduction into Eclipse Commands please see Eclipse Commands Tutorial.
2. Eclipse standard commands
2.1. Overview
Eclipse provides lots of standard commands which you can reuse. Just press on the "Browse button" while defining your commandId to see the available standard commands. For example, the screenshot shows the usage of the standard about dialog command.
The advantage of using standard commands is that you get the keybinding, icons, etc for free.
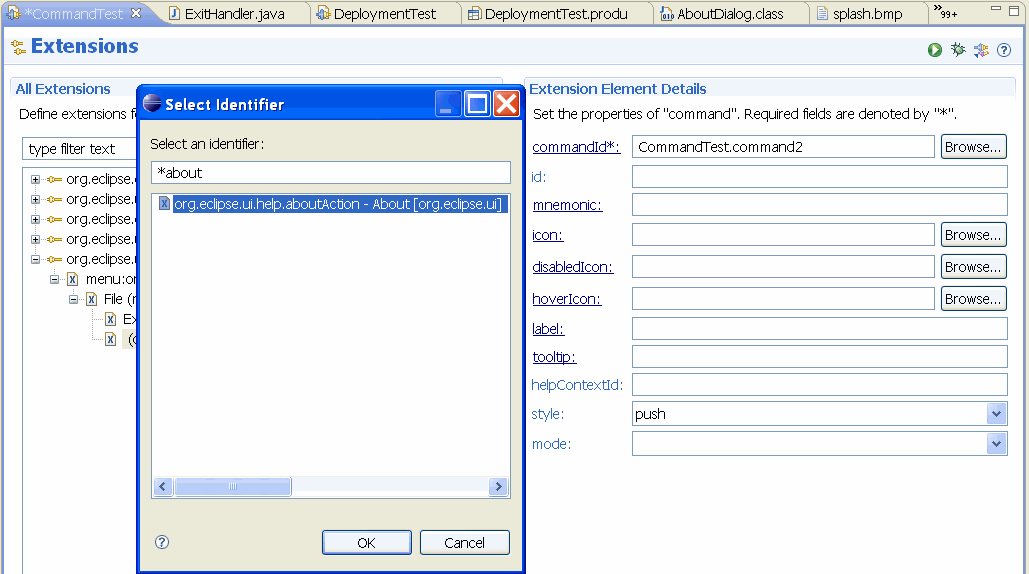
Standard commands sometimes map to actions which are contributed via ActionFactory in the class ApplicationActionBarAdvisor. If the ActionFactory returns an IAction you need to register this action. If not these commands are inactive in your menu. For example, the following made the reset perspective and welcome command active. |
// Method belongs to class ApplicationActionBarAdvisor
@Override
protected void makeActions(IWorkbenchWindow window)
{
IWorkbenchAction quickStartAction = ActionFactory.INTRO.create(window);
register(quickStartAction);
IWorkbenchAction resetView = ActionFactory.RESET_PERSPECTIVE
.create(window);
register(resetView);
}
2.2. Example
You can use standard command and define a new handler for this command, e.g., you can use the standard Eclipse delete command (org.eclipse.ui.edit.delete).
TIP: To find the ID of existing commands you can you the Eclipse Plugin Spy.
You can use the extension point "org.eclipse.ui.handlers" to define new handlers for the standard commands.
Eclipse requires you to have only one active handler at the time therefore you have to use the "activeWhen" restriction on the handler to make it more special than the standard binding. To use for example delete in several views with different handlers use "activeWhen" with the variable "activePartId" and as the value your view id. |
Create a new RCP application "de.vogella.rcp.commands.standardcommands" with the template "Hello RCP". Create a handler for the command "org.eclipse.ui.edit.delete" which display a message box. Add the command "org.eclipse.ui.edit.delete" to the menu.
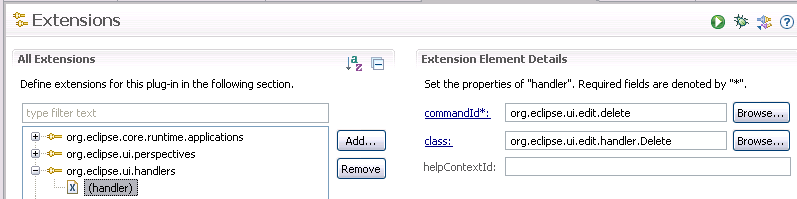
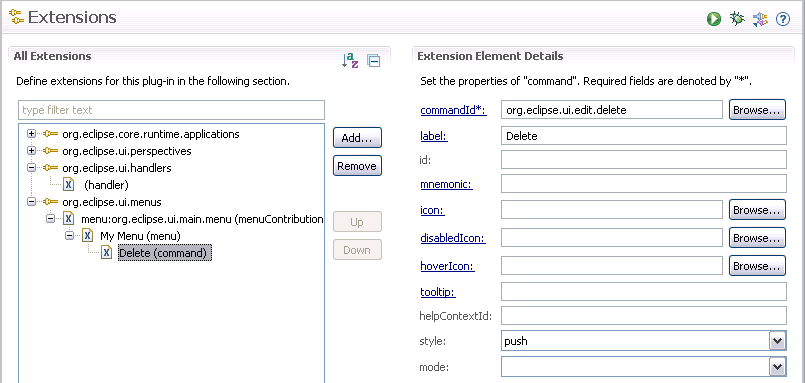
The result should look like the following.
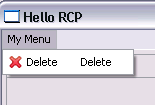
3. Calling commands directly via code
You can call a command directly. For example, if you have a command "add.command" defined you can call it via the following coding.
// From a view you get the site which allow to get the service
IHandlerService handlerService = (IHandlerService) getSite().getService(IHandlerService.class);
try {
handlerService.executeCommand("add.command", null);
} catch (Exception ex) {
throw new RuntimeException("add.command not found");
// Give message
}
}
The code above is called within a view which provides access to the site and to services. You can get the service via the WorkbenchWindow.
4. Using parameters in commands
You can define parameters in commands. Create project "de.vogella.rcp.commands.parameterfirst" using the "Hello RCP" template.
Create a command with the id "de.vogella.rcp.commands.parameterfirst.helloName" and a default handler "de.vogella.rcp.commands.parameterfirst.handler.HelloName".
Right-click on your command, select New → commandParameter
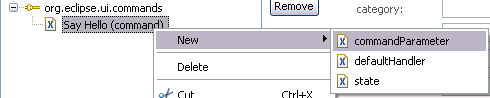
Use the following id for the parameter "de.vogella.rcp.commands.parameterfirst.commandParameter1"
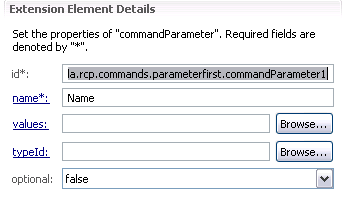
In the handler you have to evaluate the parameter.
package de.vogella.rcp.commands.parameterfirst.handler;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
import org.eclipse.jface.dialogs.MessageDialog;
import org.eclipse.ui.handlers.HandlerUtil;
public class HelloName extends AbstractHandler {
@Override
public Object execute(ExecutionEvent event) throws ExecutionException {
String name = event
.getParameter("de.vogella.rcp.commands.parameterfirst.commandParameter1");
MessageDialog.openInformation(HandlerUtil.getActiveShell(event),
"Hello", "Hello " + name);
return null;
}
}
Add this command to the menu. On the command, right-click and select New → Parameter
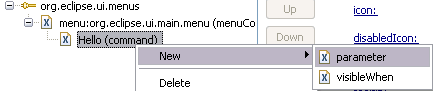
As name enter the name of the parameter, pass as value the value you want to use in your handler.
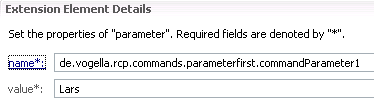
Add the same command again to the menu and pass another parameter.
If you run your application and select the menu entry the value should be used in the message box.
5. Defining commands at runtime
You can create commands at runtime. Create project "de.vogella.rcp.commands.runtimecommands" using the "Hello RCP" template.
Define a menu contribution. Maintain the class "de.vogella.rcp.commands.runtimecommands.DefineCommands" in this menu contribution.

Create the following class.
package de.vogella.rcp.commands.runtimecommands;
import org.eclipse.swt.SWT;
import org.eclipse.ui.menus.CommandContributionItem;
import org.eclipse.ui.menus.CommandContributionItemParameter;
import org.eclipse.ui.menus.ExtensionContributionFactory;
import org.eclipse.ui.menus.IContributionRoot;
import org.eclipse.ui.services.IServiceLocator;
public class DefineCommands extends ExtensionContributionFactory {
@Override
public void createContributionItems(IServiceLocator serviceLocator,
IContributionRoot additions) {
CommandContributionItemParameter p = new CommandContributionItemParameter(
serviceLocator, "",
"org.eclipse.ui.file.exit",
SWT.PUSH);
p.label = "Exit the application";
p.icon = Activator.getImageDescriptor("icons/alt_window_16.gif");
CommandContributionItem item = new CommandContributionItem(p);
item.setVisible(true);
additions.addContributionItem(item, null);
}
}
Run the example, your application should have the Exit command in the menu.
6. Links and Literature
6.2. vogella Java example code
endifdef::printrun[]
If you need more assistance we offer Online Training and Onsite training as well as consulting