Lombok. This article describes how to use Lombok for the creation of data/model objects.
1. Introduction to lombok
The official lombok website can be found here: https://projectlombok.org/
Lombok is used to reduce boilerplate code for model/data objects, e.g., it can generate
getters and setters for those object automatically by using Lombok annotations.
The easiest way is to use the @Data
annotation.
package de.vogella.jpa.simple.model;
import lombok.Data;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
@Data
public class Todo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String summary;
private String description;
}
By simply adding the @data
annotation you get all this for free:
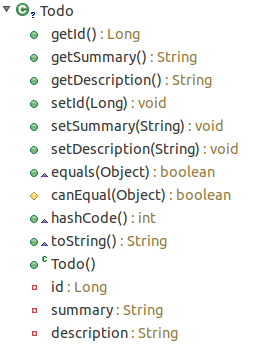
2. Enable lombok for Eclipse or Spring Tool Suite
You can download the latest lombok version from https://projectlombok.org/download.html or by using Gradle, Maven or other tools.
The lombok.jar can be copied into the root Eclipse or Spring Tool Suite folder and run like this:
java -jar lombok-1.16.12.jar
This will open a small UI, where the location of the Eclipse installation can be specified. Usually the Eclipse installation will be found automatically and lombok can be installed or updated.
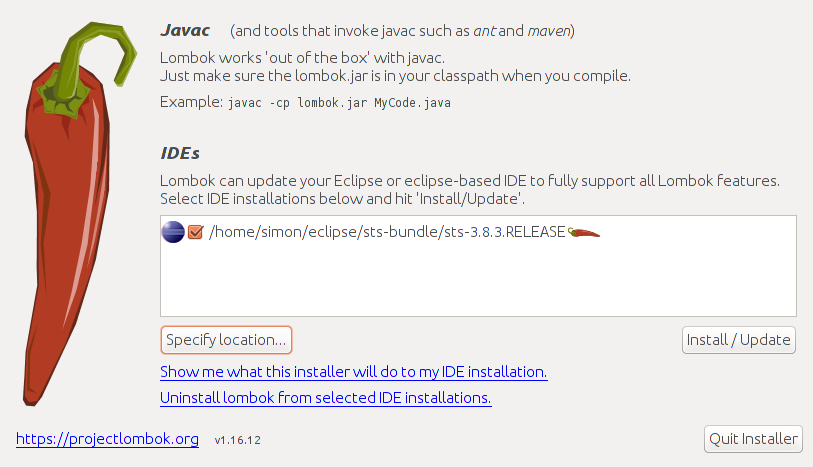
Just press the Install / Update button and restart the IDE.
Sometimes this error message is shown: ![]() Then the location of the installation has to be specified. Therefore press the Specify location… button and select the eclipse.ini or STS.ini file. ![]() |
3. Using lombok in Java projects
3.1. Using Gradle
To add lombok to the classpath of a Java Project with Gradle the following dependency has to be added:
dependencies {
compileOnly('org.projectlombok:lombok:1.16.20')
}
3.2. Using Maven
To add lombok to the classpath of a Java Project with Apache Maven the following dependency has to be added:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.20</version>
</dependency>
3.3. Doing it manually
The lombok library can also be downloaded directly from here: https://projectlombok.org/download.html and be added to the classpath.
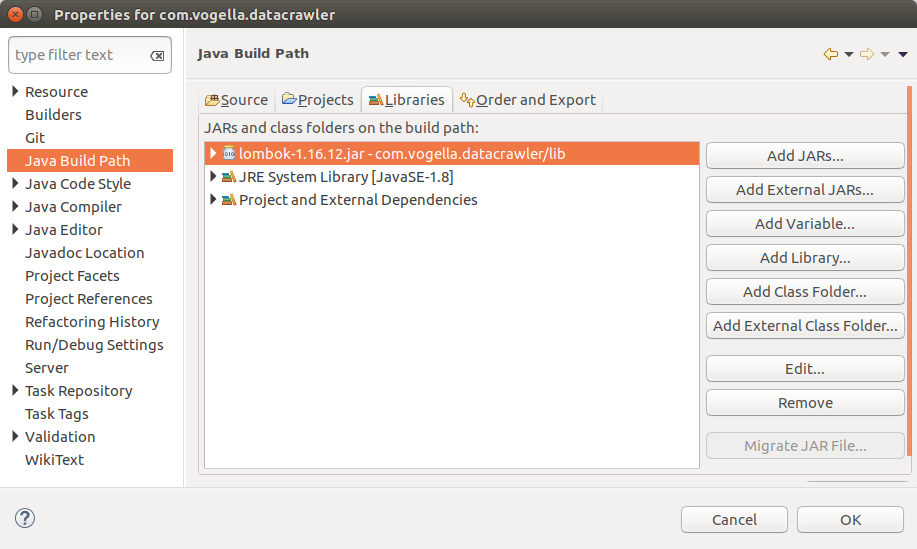
4. Lombok online resources
4.1. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting