This tutorial describes how to create and use library projects in Android.
1. Android library projects and Java libraries
Android project can use code contained in JAR files (Java libraries)
If you want to use libraries, these must only use API available in Android.
For example, the |
In addition to JAR files, the Android uses a binary distribution format called Android ARchive(AAR). The .aar bundle is the binary distribution of an Android Library Project.
An AAR is similar to a JAR file, but it can contain resources as well as compiled byte-code. A AAR file can be included in the build process of an Android application similar to a JAR file.
It is possible to create libraries modules which can be used as dependencies in Android projects. These modules allow you to store source code and Android resources which can be shared between several other Android projects.
To use a Java library (JAR file) inside your Android project, you can simple copy the JAR file into the folder called libs in your application. *.jar files in this folder are included into the compile classpath via the default build.gradle file.
2. Custom Android library modules
2.1. Using custom library modules
An Android library module can contain Java classes, Android components and resources. Only assets are not supported.
The code and resources of the library project are compiled and packaged together with the application.
Therefore a library module can be considered to be a compile-time artifact.
2.2. Creating custom Android library modules
Using library projects helps you to structure your application code.
To create a new library module in Android Studio, select Android Library
.
3. Prerequisite
The following example assumes that you have created an Android project with the com.example.android.rssfeed top level package based on the following tutorial: https://www.vogella.com/tutorials/AndroidFragments/article.html#fragments_tutorial
4. Exercise: Create an Android library module
Our library project will contain the data model and a method to get the number of instances. The library provides access to (fake) RSS data. An RSS document is an XML file which can be used to publish blog entries and news.
4.1. Create library module
For Android Studio each library is a module.
To create a new library module in Android Studio, select Android Library
.
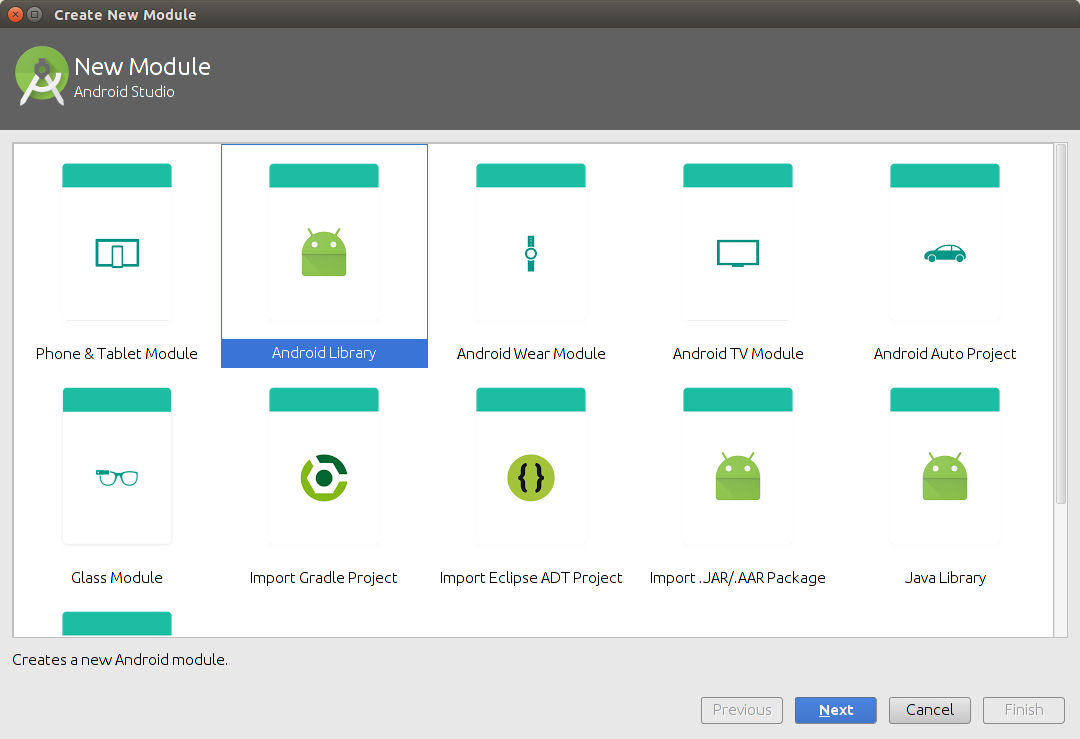
Use com.example.android.rssfeedlibrary as module name and Rssfeed Library as library name.
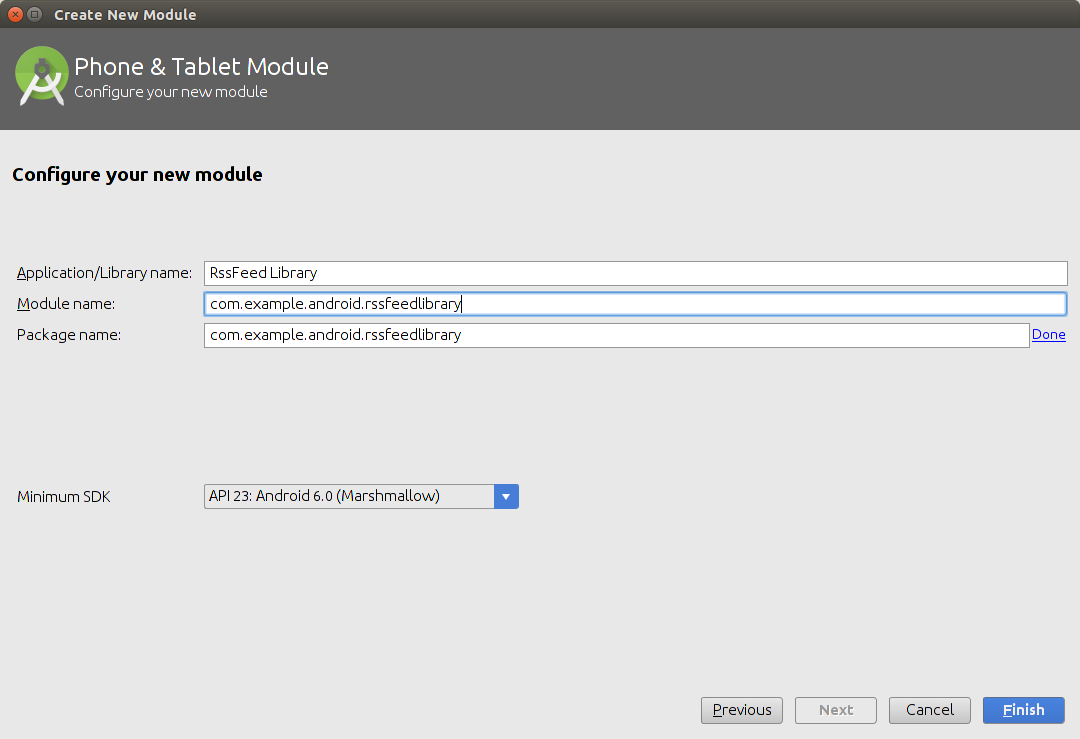
If prompted for a template select that no activity should be created. As a result Android Studio shows another module.
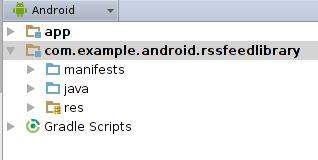
4.2. Remove generated dependency from build.gradle of the library project
Open the build.gradle
of the library project.
Delete the dependencies
closure, your library does not need any dependency and the generated dependency can cause problems for the build.
Ensure you remove |
4.3. Create the model class
Create an
RssItem
class which can store data of an RSS entry.
Generate the getters and setter, the constructor and a
toString()
method. The result should look like the following class:
package com.example.android.rssfeedlibrary;
public class RssItem {
private String pubDate;
private String description;
private String link;
private String title;
public RssItem() {
}
public RssItem(String title, String link) {
this.title = title;
this.link = link;
}
public String getPubDate() {
return pubDate;
}
public void setPubDate(String pubDate) {
this.pubDate = pubDate;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getLink() {
return link;
}
public void setLink(String link) {
this.link = link;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public String toString() {
return "RssItem [title=" + title + "]";
}
}
4.4. Create instances
Create a new class called RssFeedProvider
with a static method to return a list of RssItem
objects.
This method does currently only return test data.
package com.example.android.rssfeedlibrary;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class RssFeedProvider {
public static List<RssItem> parse(String rssFeed) {
List<RssItem> list = new ArrayList<>();
Random r = new Random();
// random number of item but at least 5
Integer number = r.nextInt(10) + 5;
for (int i = 0; i < number; i++) {
// create sample data
String s = String.valueOf(r.nextInt(1000));
RssItem item = new RssItem("Summary " + s, "Description " + s);
list.add(item);
}
return list;
}
}
4.5. Define dependency to the library project
To use the library add it as a dependency in your project select Dependencies
tab and select Module dependencies
via the + sign.
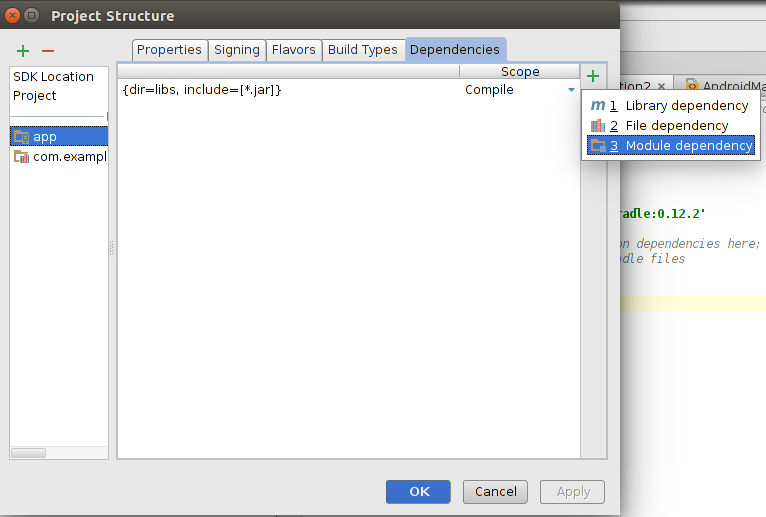
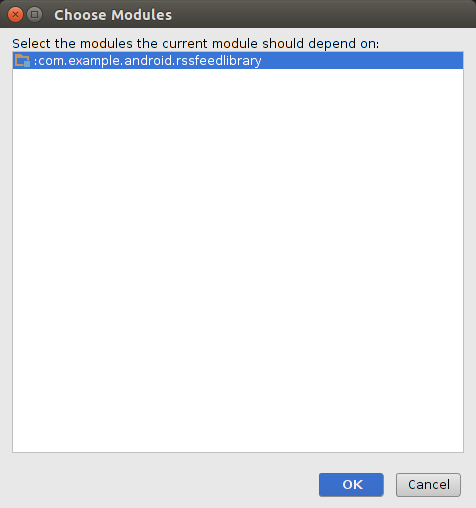
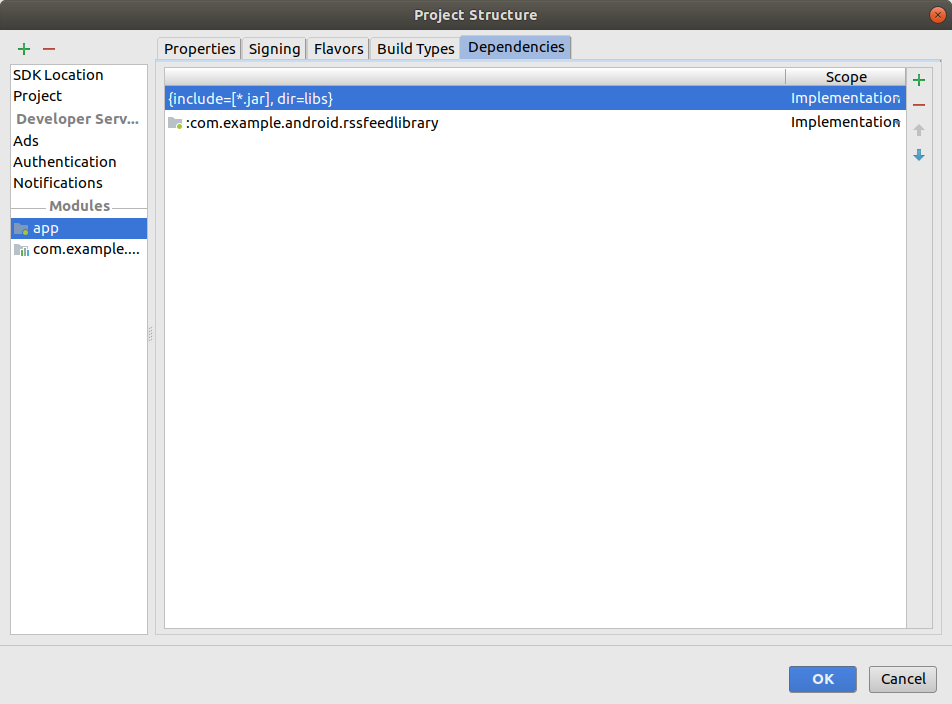
4.6. Use library project to update detailed fragments
Update the updateDetail
method in your MyListFragment
class to use the RssFeedProvider
provider.
This is only test code.
public class MyListFragment extends Fragment {
..// everything as before
// triggers update of the details fragment
public void updateDetail(String uri) { // (1)
List<RssItem> list = RssFeedProvider
.parse(uri);
String itemListAsString = list.toString();
listener.onRssItemSelected(itemListAsString);
}
1 | Updated method |
4.7. Validate implementation
Start your application and ensure that the toString
value of the list of RssItems is displayed in the DetailFragment
.
The list is currently generated randomly every time you press the button. |
5. Exercise: Deploy a library project
Create a new library project called recyclerbaseadapter
with the same top level package.
Add the following to its build.gradle file.
apply plugin: 'maven'
group = 'com.vogella.libraries'
version = '1.0'
uploadArchives {
repositories {
mavenLocal()
}
}
Create or move a MyBaseAdapter
class in this library.
Deploy it by running the gradle uploadArchives
task.
You can now define a dependency to this library, by adding mavenLocal() and using:
compile 'com.vogella.libraries:recyclerbaseadapter:1.0`
6. Android library resources
6.1. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting