This tutorial describes how to download efficiently images using Google Glide.
1. Google Glide for image processing
Every application needs to download and manage images.
Google Glide makes this easy.
To use Glide add the following dependency to your app/build.gradle file.
dependencies {
compile 'com.github.bumptech.glide:glide:3.8.0'
compile 'com.android.support:support-v4:25.3.1'
// more stuff
}
The usage is simple.
// simple usage
Glide.with(myListFragment)
.load("url").into(imageView)
// with placeholder
Glide.with(myListFragment)
.load("url").placeholder(R.drawable.loading_spinner).into(imageView)
2. Exercise - Using Glide to download images into your RecyclerView
Use Glide to download images into your RecyclerView adapter. As a first step add the Glide dependencies to your Gradle build file.
dependencies {
compile 'com.github.bumptech.glide:glide:3.8.0'
compile 'com.android.support:support-v4:25.3.1'
// more stuff
}
The Internet provides free example picture services for testing, for example:
-
http://lorempixel.com/ - Different pictures, example URL http://lorempixel.com/400/200/sports/1
-
http://placekitten.com/ - Cat pictures, example URL, example URL http://placekitten.com/g/200/200
Adjust your adapter implementation in your Recyclerview.
@Override
public void onBindViewHolder(final ViewHolder holder, final int position) {
final RssItem rssItem = rssItems.get(position);
holder.txtHeader.setText(rssItem.getTitle());
holder.txtFooter.setText(rssItem.getLink());
holder.mainLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myListFragment.updateDetail(rssItem.getLink());
}
});
// to download some random data
Random r = new Random();
int i = r.nextInt(10);
Glide.with(myListFragment).load("http://lorempixel.com/400/200/sports/"+i+"/").
into(holder.imageView);
}
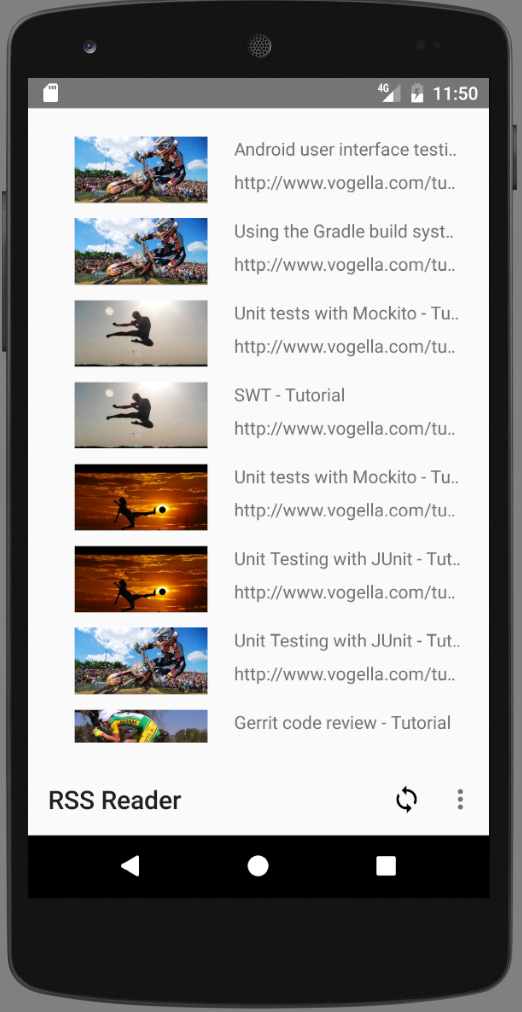
3. Links and Literature
3.2. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting