Using the toolbar in Android applications. This tutorial describes how to use the toolbar widget in your Android application. It is based on Android 6.0.
1. Introduction to the toolbar
1.1. What is the toolbar (action bar)?
The toolbar bar (formerly known as action bar) is represented as of Android 5.0 via the Toolbar
view group.
It can be freely positioined into your layout file.
It can display the activity title, icon, actions which can be triggered, additional views and other interactive items.
It can also be used for navigation in your application.
Before Android 5.0 the location of the toolbar (actionbar) was hard coded to the top of the activity. It is possible to disable the toolbar via the used theme, but the default Android themes have it enabled.
The following screenshot shows the toolbar of the Google+ Android application with interactive items and a navigation bar. On top it also indicates that the user can open a navigation bar on the side of the application.
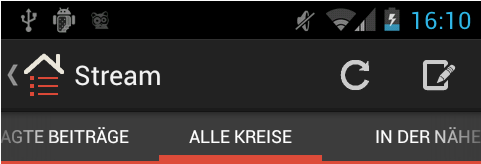
1.2. Action bar on devices lower than API 21
The toolbar has been introduced in Android 5.0 (API 21).
If you want to use the toolbar on devices with an earlier Android release you can use the downport provided by the appcompat-v7
support library.
To use the toolbar on such devices add a compile dependency to com.android.support:appcompat
to your Gradle build file.
For example:
compile `com.android.support:appcompat-v7:22.2.0`
See the following link for setting up the library v7 in your project: http://developer.android.com/tools/support-library/setup.html.
1.3. Once upon a time their was the options menu
Applications with a target SDK version less than API 11 use the options menu, if such a button is present on the device. The option menu is displayed if the user presses the Option button. The toolbar bar is superior to the options menu, as the action bar is clearly visible, while the options menu is only shown on request. In case of the options menu, the user may not recognize that options are available in the application.
2. Using the toolbar
2.1. Creating actions in the toolbar
Entries in the toolbar are typically called actions. While it is possible to create entries in the action bar via code, it is typically defined in an XML resource file.
Each menu definition is contained in a separate file in the res/menu folder.
The Android tooling automatically creates a reference to menu item entries in the R
file, so that the menu resource can be accessed.
An activity adds entries to the action bar in its onCreateOptionsMenu()
method.
The showAsAction
attribute allows you to define how the action is displayed.
For example, the ifRoom
attribute defines that the action is only displayed in the action bar if there is sufficient screen space available.
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item
android:id="@+id/action_refresh"
android:orderInCategory="100"
android:showAsAction="always"
android:icon="@drawable/ic_action_refresh"
android:title="Refresh"/>
<item
android:id="@+id/action_settings"
android:title="Settings">
</item>
</menu>
The MenuInflator
class allows to inflate actions defined in an XML file and adds them to the action bar.
MenuInflator
can get accessed via the getMenuInflator()
method from your activity.
The following example code demonstrates the creation of actions.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.mainmenu, menu);
return true;
}
While you can define the actions also in your source code, it is good practice to do this via XML files, as this results in less boilerplate code. |
2.2. Reacting to action selection
If an action is selected, the onOptionsItemSelected()
method in the corresponding activity is called.
It receives the selected action as parameter.
The usage of this method is demonstrated in the following code snippet.
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
// action with ID action_refresh was selected
case R.id.action_refresh:
Toast.makeText(this, "Refresh selected", Toast.LENGTH_SHORT)
.show();
break;
// action with ID action_settings was selected
case R.id.action_settings:
Toast.makeText(this, "Settings selected", Toast.LENGTH_SHORT)
.show();
break;
default:
break;
}
return true;
}
2.3. Search an action in the action bar
To search for a menu item in a menu you can use the findItem()
method of the Menu
class.
This method allows to search by id.
2.4. Changing the menu
The onCreateOptionsMenu()
method is only called once.
If you want to change the menu later, you have to call the invalidateOptionsMenu()
method.
Afterwards this onCreateOptionsMenu()
method is called again.
2.5. Making the toolbar context sensitive with the contextual action mode
A contextual action mode activates a temporary toolbar that overlays the application toolbar for the duration of a particular sub-task.
The contextual action mode is typically activated by selecting an item or by long clicking on it.
To implement this, call the startActionMode()
method on a viewor on your activity.
This method gets an ActionMode.Callback
object which is responsible for the life cycle of the contextual action bar.
You could also assign a context menu to a view via the registerForContextMenu(view)
method.
A context menu is also activated if the user "long presses" the view.
The onCreateContextMenu()
method is called every time a context menu is activated as the context menu is discarded after its usage.
You should prefer the contextual action mode over the usage of context menus.
2.6. Contributing to the action bar with fragments
Fragments can also contribute entries to the toolbar bar.
To do this, call setHasOptionsMenu(true)
in the onCreate()
method of the fragment.
The Android framework calls in this case the onCreateOptionsMenu()
method in the fragment class.
Here the fragment can adds menu items to the toolbar.
2.7. Changing the visibility of the toolbar bar
You can change the visibility of the toolbar at runtime. The following code demonstrates that.
ActionBar actionBar = getActionBar();
actionBar.hide();
// more stuff here...
actionBar.show();
You can also change the text which is displayed alongside the application icon at runtime. The following example shows that.
ActionBar actionBar = getActionBar();
actionBar.setSubtitle("mytest");
actionBar.setTitle("vogella.com");
2.8. Assigning a Drawable
You also add a Drawable
to the action bar as background via the ActionBar.setBackgroundDrawable()
method.
The toolbar scales the image. Therefore it is best practice to provide a scalable drawable, e.g., a 9-patch or XML drawable.
As of Android 4.2 the background of the action bar can also be animated via an AnimationDrawable
.
2.9. Dimming the navigation buttons
You can also dim the software navigation button in your Android application to have more space available. If the user touches the button of the screen, the navigation button is automatically shown again.
Dimming the navigation buttons is demonstrated by the following code snippet.
getWindow().
getDecorView().
setSystemUiVisibility(View.SYSTEM_UI_FLAG_HIDE_NAVIGATION);
The following screenshots show an application with and without the navigation buttons.
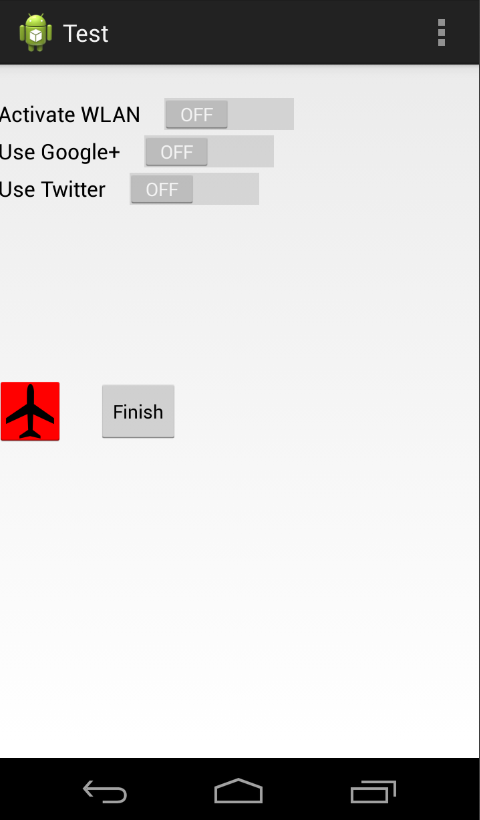
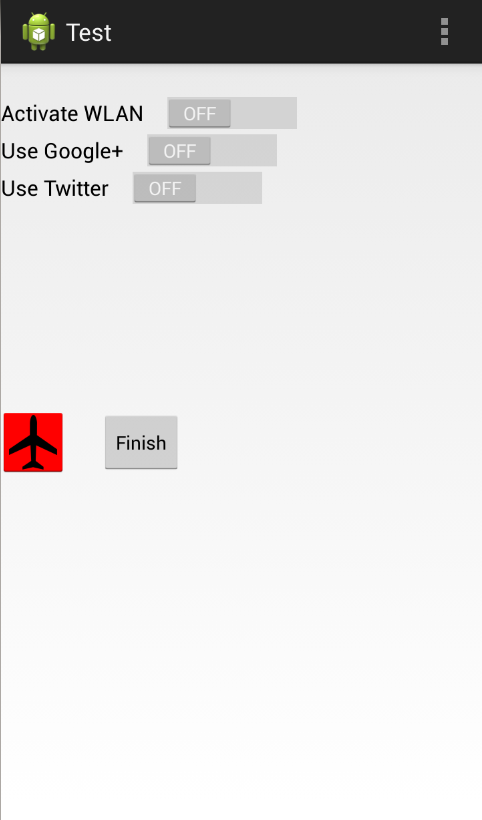
2.10. Using immersive full screen mode
As of Android 4.4 (API 19) you can put your application into full screen mode. The first time this happens the system displays the user the info that he can restore the system bars with a downward swipe along the region where the system bars normally appear.
For example the following method also to put an activity into full screen mode.
// This method hides the system bars and resize the content
private void hideSystemUI() {
getWindow().getDecorView().setSystemUiVisibility(
View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN
| View.SYSTEM_UI_FLAG_HIDE_NAVIGATION // hide nav bar
| View.SYSTEM_UI_FLAG_FULLSCREEN // hide status bar
// remove the following flag for version < API 19
| View.SYSTEM_UI_FLAG_IMMERSIVE
);
}
2.11. Enabling the split toolbar
You can define that the toolbar should be automatically split by the system if not enough space is available.
You can activate this via the android:uiOptions="SplitActionBarWhenNarrow"
parameter in the declaration of your application activity in the AndroidManifest.xml file.
If this option is activated, Android has the option to split the toolbar. Whether to split is decided by the system at runtime. |
3. Exercise: Using the contextual action mode
3.1. Target
In this exercise you add a contextual action mode to one of your existing applications.
3.2. Create menu resource in the res/menu folder
For this, create a new menu XML resource with the actionmode.xml file name. See <<androidstudio_createmenu" /> for details.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/action_share"
android:title="Share it"
>
</item>
</menu>
3.3. Adjust your coding
Extend your fragment interface.
public class MyListFragment extends Fragment {
public void goToActionMode(RssItem item) {
listener.goToActionMode(item);
}
public interface OnItemSelectedListener {
public void onRssItemSelected(String link);
public void goToActionMode(RssItem item);
}
Change your activity to implement this new method and also the ActionMode.Callback
callback as demonstrated in the following example code.
package com.example.android.rssreader;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.ActionMode;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.widget.Toast;
import android.widget.Toolbar;
import com.example.android.rssfeedlibrary.RssItem;
public class RssfeedActivity extends Activity
implements MyListFragment.OnItemSelectedListener,
ActionMode.Callback {
private RssItem selectedRssItem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar tb = (Toolbar) findViewById(R.id.toolbar);
setActionBar(tb);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
Toolbar tb = (Toolbar) findViewById(R.id.toolbar);
tb.inflateMenu(R.menu.mainmenu);
tb.setOnMenuItemClickListener(
new Toolbar.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
return onOptionsItemSelected(item);
}
});
return true;
}
//NEW
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_refresh:
MyListFragment fragment = (MyListFragment) getFragmentManager()
.findFragmentById(R.id.listFragment);
fragment.updateListContent();
break;
case R.id.action_settings:
Intent intent = new Intent(this, MyPreferences.class);
startActivity(intent);
Toast.makeText(this, "Action Settings selected", Toast.LENGTH_SHORT)
.show();
break;
default:
break;
}
return true;
}
@Override
public void onRssItemSelected(String link) {
if (getResources().getBoolean(R.bool.twoPaneMode)) {
DetailFragment fragment = (DetailFragment) getFragmentManager()
.findFragmentById(R.id.detailFragment);
fragment.setText(link);
} else {
Intent intent = new Intent(getApplicationContext(),
DetailActivity.class);
intent.putExtra(DetailActivity.EXTRA_URL, link);
startActivity(intent);
}
}
@Override
public void showContextMenu(RssItem item) {
this.selectedRssItem = item;
startActionMode(this);
}
@Override
public void goToActionMode(RssItem item) {
this.selectedRssItem = item;
startActionMode(this);
}
@Override
public boolean onCreateActionMode(ActionMode mode, Menu menu) {
MenuInflater inflater = mode.getMenuInflater();
inflater.inflate(R.menu.actionmode, menu);
return true;
}
@Override
public boolean onPrepareActionMode(ActionMode mode, Menu menu) {
return false;
}
@Override
public boolean onActionItemClicked(ActionMode mode, MenuItem item) {
Intent intent = new Intent(Intent.ACTION_SEND);
intent.putExtra(Intent.EXTRA_TEXT, "I found this interesting link" +
selectedRssItem.getLink());
intent.setType("text/plain");
startActivity(intent);
mode.finish(); // Action picked, so close the CAB
selectedRssItem = null;
return true;
}
@Override
public void onDestroyActionMode(ActionMode mode) {
}
}
In your adapter implement a LongClickListener
which triggers the contextual action mode.
package com.example.android.rssreader;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.bumptech.glide.Glide;
import com.example.android.rssfeedlibrary.RssItem;
import java.util.List;
import java.util.Random;
public class RssItemAdapter
extends RecyclerView.Adapter<RssItemAdapter.ViewHolder> {
private List<RssItem> rssItems;
private MyListFragment myListFragment;
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View v= null;
v = LayoutInflater.
from(parent.getContext()).
inflate(R.layout.rowlayout, parent, false);
return new ViewHolder(v);
}
public static class ViewHolder extends RecyclerView.ViewHolder {
public View mainLayout;
public TextView txtHeader;
public TextView txtFooter;
public ImageView imageView;
public ViewHolder(View v) {
super(v);
mainLayout = v;
txtHeader = (TextView) v.findViewById(R.id.rsstitle);
txtFooter = (TextView) v.findViewById(R.id.rssurl);
imageView = (ImageView) v.findViewById(R.id.icon);
}
}
@Override
public void onBindViewHolder(final ViewHolder holder, final int position) {
final RssItem rssItem = rssItems.get(position);
holder.txtHeader.setText(rssItem.getTitle());
holder.txtFooter.setText(rssItem.getLink());
holder.mainLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myListFragment.updateDetail(rssItem.getLink());
}
});
holder.mainLayout.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
myListFragment.goToActionMode(rssItem);
return true;
}
});
}
@Override
public int getItemCount() {
return rssItems.size();
}
public RssItemAdapter(List<RssItem> rssItems, MyListFragment myListFragment) {
this.rssItems = rssItems;
this.myListFragment = myListFragment;
}
}
4. Making the action bar dynamic
4.1. Custom views in the action bar
You can also add a custom view to the action bar, for example, a button or a text field.
For this you use the setCustomView
method of the ActionView
class.
You also have to enable the display of custom views via the setDisplayOptions()
method by passing in the ActionBar.DISPLAY_SHOW_CUSTOM
flag.
For example, you can define a layout file which contains a EditText
element.
<?xml version="1.0" encoding="utf-8"?>
<EditText xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/searchfield"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:inputType="textFilter" >
</EditText>
This layout can be assigned in an activity to the action bar via the following code. The example code also attaches a listener to the custom view.
package com.vogella.android.actionbar.customviews;
import android.app.ActionBar;
import android.app.Activity;
import android.os.Bundle;
import android.view.KeyEvent;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.TextView.OnEditorActionListener;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActionBar actionBar = getActionBar();
// add the custom view to the action bar
actionBar.setCustomView(R.layout.actionbar_view);
EditText search = (EditText) actionBar.getCustomView().findViewById(
R.id.searchfield);
search.setOnEditorActionListener(new OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId,
KeyEvent event) {
Toast.makeText(MainActivity.this, "Search triggered",
Toast.LENGTH_LONG).show();
return false;
}
});
actionBar.setDisplayOptions(ActionBar.DISPLAY_SHOW_CUSTOM
| ActionBar.DISPLAY_SHOW_HOME);
}
}
4.2. Action view
An action view _ is a widget that appears in the _action bar as a substitute for an action item’s button.
You can, for example, use this feature to replace an action item with a ProgressBar
view.
An action view for an action can be defined via the android:actionLayout
or android:actionViewClass
attribute to specify either a layout resource or widget class to use.
This replacement is depicted in the following screenshots.
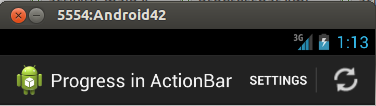
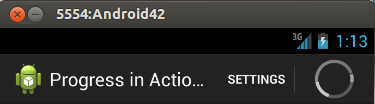
The following activity replaces the icon at runtime with an action view which contains a ProgressBar
view.
package com.vogella.android.actionbar.progress;
import android.app.ActionBar;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
public class MainActivity extends Activity {
private MenuItem menuItem;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ActionBar actionBar = getActionBar();
actionBar.setDisplayOptions(ActionBar.DISPLAY_SHOW_HOME
| ActionBar.DISPLAY_SHOW_TITLE | ActionBar.DISPLAY_SHOW_CUSTOM);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.menu_load:
menuItem = item;
menuItem.setActionView(R.layout.progressbar);
menuItem.expandActionView();
TestTask task = new TestTask();
task.execute("test");
break;
default:
break;
}
return true;
}
private class TestTask extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
// Simulate something long running
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(String result) {
menuItem.collapseActionView();
menuItem.setActionView(null);
}
};
}
The following code shows the layout used for the action view.
<?xml version="1.0" encoding="utf-8"?>
<ProgressBar xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/progressBar2"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
</ProgressBar>
The following code shows the XML files for the menu.
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item
android:id="@+id/menu_settings"
android:orderInCategory="100"
android:showAsAction="always"
android:title="Settings"
/>
<item
android:id="@+id/menu_load"
android:icon="@drawable/navigation_refresh"
android:orderInCategory="200"
android:showAsAction="always"
android:title="Load"/>
</menu>
5. Action provider
5.1. What is an action provider?
An action provider defines rich menu interaction in a single component. It can generate action views, which are used in the action bar, dynamically populate sub-menus of an action and handle default action invocations.
The base class for an action provider is the ActionProvider
class.
Currently the Android platform provides two action providers: the MediaRouteActionProvider
and the ShareActionProvider
.
5.2. Example: usage of the ShareActionProvider
The following demonstrates the usage of the ShareActionProvider
.
This action provider allows you to grab selected content from applications which have registered the Intent.ACTION_SEND
intent.
To use ShareActionProvider
, you have to define a special menu entry for it and assign an intent which contains the sharing data to it.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:id="@+id/menu_share"
android:title="Share"
android:showAsAction="ifRoom"
android:actionProviderClass="android.widget.ShareActionProvider" />
<item
android:id="@+id/item1"
android:showAsAction="ifRoom"
android:title="More entries...">
</item>
</menu>
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
// Get the ActionProvider for later usage
provider = (ShareActionProvider) menu.findItem(R.id.menu_share)
.getActionProvider();
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.menu_share:
doShare();
break;
default:
break;
}
return true;
}
public void doShare() {
// populate the share intent with data
Intent intent = new Intent(Intent.ACTION_SEND);
intent.setType("text/plain");
intent.putExtra(Intent.EXTRA_TEXT, "This is a message for you");
provider.setShareIntent(intent);
}
6. Navigation via the application icon
6.1. Application icon as home
The action bar shows an icon of your application. This is called the home icon. You can assign an action to this icon. The recommendation is to return to the main activity in your program if the user selects this icon.
If the action is selected, the onOptionsItemSelected()
method is called with an action which has the android.R.id.home
ID.
Before Android 4.1, you had to use the android.R.id.home
ID in the onOptionMenuItemSelected()
method and enable the selection of the home button.
This is demonstrated by the following code in which the SecondActivity
activity defines the MainActivity
as home.
package com.vogella.android.actionbar.homebutton;
import android.os.Bundle;
import android.app.ActionBar;
import android.app.Activity;
import android.content.Intent;
import android.view.Menu;
import android.view.MenuItem;
public class SecondActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// enable the home button
ActionBar actionBar = getActionBar();
actionBar.setHomeButtonEnabled(true);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
Intent intent = new Intent(this, MainActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
startActivity(intent);
break;
// Something else
case R.id.action_settings:
intent = new Intent(this, ThirdActivity.class);
startActivity(intent);
default:
break;
}
return super.onOptionsItemSelected(item);
}
}
As of Android 4.1 you can simply set the
|
6.2. Application icon as Up button
You can use the application icon also as Up button, e.g., to go to the parent activity of the current activity. The back button on the device always returns to the previous activity.
Both can be different, for example, if the user started the option to write an email from the home screen, the back button will return the user to the home screen while the Up button would return the user to the activity which shows an overview of all emails.
To enable the Up display, you can use the following code snippet in your activity.
actionBar.setDisplayUseLogoEnabled(false);
actionBar.setDisplayHomeAsUpEnabled(true);
This snippet only enables the Up display on your home icon.
You need to implement the correct behavior in your activity in the onOptionsItemSelected() method.
The corresponding action still has the android.R.id.home ID.
|
The difference between Up and the Back button can be confusing for the end user. If you decide to implement Up, in your application, it is recommended to perform some end user testing to see if the Up implementation is intuitive for them or not. |
7. Exercise: Using the toolbar
This exercise demonstrates how to use the toolbar widget in your Android application.
It is based on the fragment tutorial which can be found under: https:///www.vogella.com/tutorials/AndroidFragments/article.html You have to finish this tutorial before continuing this one.
8. Exercise: Add a toolbar to your application
8.1. Add a refresh icon
Continue to use the RSS Reader project. Create a new drawable called ic_refresh via the
menu entry. Select a fitting image from the provided example images.
If the fill color does not fit to your styling, open the created drawable and adjust the color. |
8.2. Add menu resources
Create a new XML resource for your menu called mainmenu.xml in your menu resource folder (create one, if the folder does not exist). The resulting XML file should look similar to the following listing.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:title="Refresh"
android:icon="@drawable/ic_refresh"
android:id="@+id/action_refresh"
android:showAsAction="ifRoom" />
<item
android:id="@+id/action_settings"
android:title="Settings"
android:showAsAction="never"
>
</item>
<item
android:id="@+id/action_network"
android:title="Network Setting"
android:showAsAction="never"
>
</item>
</menu>
8.3. Turn off the default action bar from the styling
Disable the default action bar one via the res/values/styles.xml file.
The following styling setting assumes that |
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="android:Theme.Material.Light.NoActionBar">
<!-- Customize your theme here. -->
</style>
</resources>
8.4. Add a toolbar view to your activity layout
Change the layout files of your RssfeedActivity
activity to also contain a toolbar entry.
For this you need to wrap your existing layout definition into another LinearLayout
and add the toolbar to this second layout file.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".RssfeedActivity"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:orientation="horizontal"
android:layout_weight="1"
>
<fragment
android:id="@+id/listFragment"
class="com.example.android.rssreader.MyListFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
tools:layout="@layout/fragment_rsslist_overview"/>
<fragment
android:id="@+id/detailFragment"
class="com.example.android.rssreader.DetailFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="2"
tools:layout="@layout/fragment_rssitem_detail" />
</LinearLayout>
<Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
</Toolbar>
</LinearLayout>
Do the same for the layout for the portrait mode.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".RssfeedActivity"
android:orientation="vertical">
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:orientation="horizontal"
>
</FrameLayout>
<Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
</Toolbar>
</LinearLayout>
8.5. Adjust your activity
Change your RssfeedActivity
class to the following code, to configure the toolbar view.
package com.example.android.rssreader;
import android.app.Activity;
import android.app.Fragment;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.FrameLayout;
import android.widget.Toast;
import android.widget.Toolbar;
public class RssfeedActivity extends Activity implements
MyListFragment.OnItemSelectedListener {
// as before
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// (1)
Toolbar tb = (Toolbar) findViewById(R.id.toolbar);
setActionBar(tb);
// more code as before
}
// (2)
@Override
public boolean onCreateOptionsMenu(Menu menu) {
Toolbar tb = (Toolbar) findViewById(R.id.toolbar);
tb.inflateMenu(R.menu.mainmenu);
tb.setOnMenuItemClickListener(
new Toolbar.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
return onOptionsItemSelected(item);
}
});
return true;
}
// (3)
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_refresh:
Toast.makeText(this, "Action Refresh selected", Toast.LENGTH_SHORT).show();
return true;
case R.id.action_settings:
Toast.makeText(this, "Action Settings selected", Toast.LENGTH_SHORT).show();
return true;
case R.id.action_network:
Toast.makeText(this, "Action Network selected", Toast.LENGTH_SHORT).show();
return true;
default:
break;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onRssItemSelected(String link) {
// more code as before
}
}
8.6. Run the application and test the toolbar
Run your application and validate that you can select both of your actions. Ensure that a Toast is displayed if you select the different entries.
If your device or emulator has an Option menu button, you will not see the overflow menu. You need to press the Option key to see the actions which are part of the overflow menu. |
8.7. Trigger an intent via the toolbar
If the action_network
action is selected, trigger the intent to control the network connectivity.
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
... as before
case R.id.action_network:
Intent wirelessIntent = new Intent("android.settings.WIRELESS_SETTINGS");
startActivity(wirelessIntent);
return true;
...as before
}
9. Trigger a method in your fragments via the toolbar
In this exercise you call a method in your list fragment based on the toolbar selection. It continues to use the RSS Reader project.
Create a updateListContent
method in MyListFragment
.
public void updateListContent() {
Toast.makeText(getContext(), "updateListContent called in MyListFragment", Toast.LENGTH_SHORT).show();
}
In your RssfeedActivity
class, call the new method, if the action_refresh
action is selected.
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_refresh:
if (getResources().getBoolean(R.bool.twoPaneMode)) {
MyListFragment fragment = (MyListFragment) getFragmentManager().findFragmentById(R.id.listFragment);
fragment.updateListContent();
} else {
Fragment fragmentById = getFragmentManager().findFragmentById(R.id.fragment_container);
if (fragmentById instanceof MyListFragment) {
MyListFragment fragment = (MyListFragment) fragmentById;
fragment.updateListContent();
}
}
return true;
// more code....
}
10. Android ActionBar Resources
http://www.grokkingandroid.com/adding-action-items-from-within-fragments Tutorial about Actionbar]
If you need more assistance we offer Online Training and Onsite training as well as consulting