JPA with Eclipselink. This tutorial explains how to use the Java persistence API. It uses EclipseLink, the reference implementation for the Java Persistence API (JPA).
1. Java Persistence API (JPA)
1.1. Overview
Mapping Java objects to database tables and vice versa is called Object-relational mapping (ORM). The Java Persistence API (JPA) is one possible approach to ORM. Via JPA the developer can map, store, update and retrieve data from relational databases to Java objects and vice versa. JPA can be used in Java-EE and Java-SE applications.
JPA is a specification and several implementations are available. Popular implementations are Hibernate, EclipseLink and Apache OpenJPA. The reference implementation of JPA is EclipseLink.
JPA permits the developer to work directly with objects rather than with SQL statements. The JPA implementation is typically called persistence provider.
The mapping between Java objects and database tables is defined via persistence metadata. The JPA provider will use the persistence metadata information to perform the correct database operations.
JPA metadata is typically defined via annotations in the Java class. Alternatively, the metadata can be defined via XML or a combination of both. A XML configuration overwrites the annotations.
The following description is based on the usage of annotations.
JPA defines a SQL-like Query language for static and dynamic queries.
Most JPA persistence providers offer the option to create the database schema automatically based on the metadata.
1.2. Entity
A class which should be persisted in a database it must be annotated with javax.persistence.Entity
.
Such a class is called Entity
.
JPA uses a database table for every entity.
Persisted instances of the class will be represented as one row in the table.
All entity classes must define a primary key, must have a non-arg constructor and or not allowed to be final. Keys can be a single field or a combination of fields.
JPA allows to auto-generate the primary key
in the database via the
@GeneratedValue
annotation.
By default, the table name corresponds to the class name. You can change this with the addition to the annotation @Table(name="NEWTABLENAME").
1.3. Persistence of fields
The fields of the Entity will be saved in the database. JPA can use
either your instance variables (fields) or the
corresponding getters
and setters to access the fields. You are not allowed to mix both
methods. If you want to use the
setter and getter methods the Java
class must follow the Java Bean naming conventions. JPA persists per
default all
fields of an Entity, if fields should not be saved they
must be marked with
@Transient
.
By default each field is mapped to a column with the name of
the
field. You can change the default name via
@Column
(name="newColumnName").
The following annotations can be used.
@Id | Identifies the unique ID of the database entry |
---|---|
@GeneratedValue |
Together with an ID this annotation defines that this value is generated automatically. |
@Transient |
Field will not be saved in database |
1.4. Relationship Mapping
JPA allows to define relationships between classes, e.g. it can be defined that a class is part of another class (containment). Classes can have one to one, one to many, many to one, and many to many relationships with other classes.
A relationship can be bidirectional or unidirectional, e.g. in a bidirectional relationship both classes store a reference to each other while in an unidirectional case only one class has a reference to the other class. Within a bidirectional relationship you need to specify the owning side of this relationship in the other class with the attribute "mappedBy", e.g. @ManyToMany(mappedBy="attributeOfTheOwningClass".
Relationship annotations:
-
@OneToOne
-
@OneToMany
-
@ManyToOne
-
@ManyToMany
1.5. Entity Manager
The entity manager
javax.persistence.EntityManager
provides the operations from and to the database, e.g.
find objects,
persists them, remove objects from the
database,
etc.
In a JavaEE application the entity manager is automatically inserted in the web application. Outside JavaEE you need to manage the entity manager yourself.
Entities which are managed by an Entity Manager will automatically propagate these changes to the database (if this happens within a commit statement). If the Entity Manager is closed (via close()) then the managed entities are in a detached state. If synchronize them again with the database a Entity Manager provides the merge() method.
The persistence context describes all Entities of one Entity manager.
1.6. Persistence units
The
EntityManager
is created by the
EntityManagerFactory
which is configured by the persistence unit. The persistence unit is
described via the
persistence.xml
file in the
META-INF
directory of
the source folder. A set of entities
which are logical
connected will
be grouped via a persistence unit.
The persistence.xml
file defines the connection data to the database, e.g. the driver, the user and the password.
2. EclipseLink
This tutorial will use EclipseLink as JPA implementation. EclipseLink supports several Java standards:
-
Java Persistence (JPA) 2.0 - JSR 317
-
Java Architecture for XML Binding (JAXB) 2.2 - JSR 222
-
Service Data Objects (SDO) 2.1.1 - JSR 235
This tutorial covers the usage of the JPA functionality.
3. Installation
3.1. EclipseLink
Download the "EclipseLink Installer Zip" implementation from the EclipseLink Download Site.
The download contains several jars. We need the following jars:
-
eclipselink.jar
-
javax.persistence_*.jar
3.2. Derby Database
The example later will be using Apache Derby as a database. Download Derby from http://db.apache.org/derby/ From this tutorial we will need the "derby.jar". For details on Derby (which is not required for this tutorial) please see Apache Derby.
4. Exercise: Usage of JPA in an Java application
4.1. Project and Entity
Create a Java project "de.vogella.jpa.simple". Create a folder "lib" and place the required JPA jars and derby.jar into this folder. Add the libs to the project classpath.
Afterwards create the package "de.vogella.jpa.simple.model" and create the following classes.
package de.vogella.jpa.simple.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Todo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String summary;
private String description;
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public String toString() {
return "Todo [summary=" + summary + ", description=" + description
+ "]";
}
}
4.2. Persistence Unit
Create a directory "META-INF" in your "src" folder and create the file "persistence.xml". This examples uses EclipseLink specific flags for example via the parameter "eclipselink.ddl-generation" you specify that the database scheme will be automatically dropped and created.
<?xml version="1.0" encoding="UTF-8" ?>
<persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd"
version="2.0" xmlns="http://java.sun.com/xml/ns/persistence">
<persistence-unit name="todos" transaction-type="RESOURCE_LOCAL">
<class>de.vogella.jpa.simple.model.Todo</class>
<properties>
<property name="javax.persistence.jdbc.driver" value="org.apache.derby.jdbc.EmbeddedDriver" />
<property name="javax.persistence.jdbc.url"
value="jdbc:derby:/home/vogella/databases/simpleDb;create=true" />
<property name="javax.persistence.jdbc.user" value="test" />
<property name="javax.persistence.jdbc.password" value="test" />
<!-- EclipseLink should create the database schema automatically -->
<property name="eclipselink.ddl-generation" value="create-tables" />
<property name="eclipselink.ddl-generation.output-mode" value="database" />
</properties>
</persistence-unit>
</persistence>
The database specified via "javax.persistence.jdbc.url" will be automatically created by the Derby Driver. You may want to adjust the path, it currently is based on Linux notations and points to my home directory on my Linux system.
To see the SQL generated for the databases set eclipselink.ddl-generation.output-mode value from "database" to "sql-script" or "both". Two files will get generated 'createDDL.jdbc' and 'dropDDL.jdbc'
4.3. Test your installation
Create the following Main class which will create a new entry every time it is called. After the first call you need to remove the property "eclipselink.ddl-generation" from persistence.xml otherwise you will receive an error as EclipseLink tries to create the database scheme again. Alternative you could set the property to "drop-and-create-tables" but this would drop your database schema at every run.
package de.vogella.jpa.simple.main;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
import javax.persistence.Query;
import de.vogella.jpa.simple.model.Todo;
public class Main {
private static final String PERSISTENCE_UNIT_NAME = "todos";
private static EntityManagerFactory factory;
public static void main(String[] args) {
factory = Persistence.createEntityManagerFactory(PERSISTENCE_UNIT_NAME);
EntityManager em = factory.createEntityManager();
// read the existing entries and write to console
Query q = em.createQuery("select t from Todo t");
List<Todo> todoList = q.getResultList();
for (Todo todo : todoList) {
System.out.println(todo);
}
System.out.println("Size: " + todoList.size());
// create new todo
em.getTransaction().begin();
Todo todo = new Todo();
todo.setSummary("This is a test");
todo.setDescription("This is a test");
em.persist(todo);
em.getTransaction().commit();
em.close();
}
}
Run you program several times to see that the database is filled.
5. Using lombok for entity classes
In order to avoid writing too much boilerplate code for the
entity
classes you can use lombok, which automatically
generates appropriate getters, setters,
toString()
and
hashCode()
methods for the fields in a Java
class.
So the code of the Todo
can be reduced to this:
package de.vogella.jpa.simple.model;
import lombok.Data;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
@Data
public class Todo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String summary;
private String description;
}
See https://www.vogella.com/tutorials/Lombok/article.html for further information and more configuration possibilities of lombok.
6. Relationship example
Create a Java project called "de.vogella.jpa.eclipselink", create again a folder "lib" and place the required JPA jars and derby.jar into this folder.
Create the de.vogella.jpa.eclipselink.model
package and the following classes.
package de.vogella.jpa.eclipselink.model;
import java.util.ArrayList;
import java.util.List;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
@Entity
public class Family {
@Id
@GeneratedValue(strategy = GenerationType.TABLE)
private int id;
private String description;
@OneToMany(mappedBy = "family")
private final List<Person> members = new ArrayList<Person>();
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public List<Person> getMembers() {
return members;
}
}
package de.vogella.jpa.eclipselink.model;
import java.util.ArrayList;
import java.util.List;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.Transient;
@Entity
public class Person {
@Id
@GeneratedValue(strategy = GenerationType.TABLE)
private String id;
private String firstName;
private String lastName;
private Family family;
private String nonsenseField = "";
private List<Job> jobList = new ArrayList<Job>();
public String getId() {
return id;
}
public void setId(String Id) {
this.id = Id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
// Leave the standard column name of the table
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@ManyToOne
public Family getFamily() {
return family;
}
public void setFamily(Family family) {
this.family = family;
}
@Transient
public String getNonsenseField() {
return nonsenseField;
}
public void setNonsenseField(String nonsenseField) {
this.nonsenseField = nonsenseField;
}
@OneToMany
public List<Job> getJobList() {
return this.jobList;
}
public void setJobList(List<Job> nickName) {
this.jobList = nickName;
}
}
package de.vogella.jpa.eclipselink.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Job {
@Id
@GeneratedValue(strategy = GenerationType.TABLE)
private int id;
private double salery;
private String jobDescr;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public double getSalery() {
return salery;
}
public void setSalery(double salery) {
this.salery = salery;
}
public String getJobDescr() {
return jobDescr;
}
public void setJobDescr(String jobDescr) {
this.jobDescr = jobDescr;
}
}
Create the file "persistence.xml" in "src/META-INF". Remember to change the path to the database.
<?xml version="1.0" encoding="UTF-8" ?>
<persistence xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_2_0.xsd"
version="2.0" xmlns="http://java.sun.com/xml/ns/persistence">
<persistence-unit name="people" transaction-type="RESOURCE_LOCAL">
<class>de.vogella.jpa.eclipselink.model.Person</class>
<class>de.vogella.jpa.eclipselink.model.Family</class>
<class>de.vogella.jpa.eclipselink.model.Job</class>
<properties>
<property name="javax.persistence.jdbc.driver" value="org.apache.derby.jdbc.EmbeddedDriver" />
<property name="javax.persistence.jdbc.url"
value="jdbc:derby:/home/vogella/databases/relationsshipDb;create=true" />
<property name="javax.persistence.jdbc.user" value="test" />
<property name="javax.persistence.jdbc.password" value="test" />
<!-- EclipseLink should create the database schema automatically -->
<property name="eclipselink.ddl-generation" value="create-tables" />
<property name="eclipselink.ddl-generation.output-mode"
value="database" />
</properties>
</persistence-unit>
</persistence>
The following check is implemented as a JUnit Test. For details please see JUnit Tutorial. The setup() method will create a few test entries. After the test entries are created, they will be read and the one field of the entries is changed and saved to the database.
package de.vogella.jpa.eclipselink.main;
import static org.junit.Assert.assertTrue;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
import javax.persistence.Query;
import org.junit.Before;
import org.junit.Test;
import de.vogella.jpa.eclipselink.model.Family;
import de.vogella.jpa.eclipselink.model.Person;
public class JpaTest {
private static final String PERSISTENCE_UNIT_NAME = "people";
private EntityManagerFactory factory;
@Before
public void setUp() throws Exception {
factory = Persistence.createEntityManagerFactory(PERSISTENCE_UNIT_NAME);
EntityManager em = factory.createEntityManager();
// Begin a new local transaction so that we can persist a new entity
em.getTransaction().begin();
// read the existing entries
Query q = em.createQuery("select m from Person m");
// Persons should be empty
// do we have entries?
boolean createNewEntries = (q.getResultList().size() == 0);
// No, so lets create new entries
if (createNewEntries) {
assertTrue(q.getResultList().size() == 0);
Family family = new Family();
family.setDescription("Family for the Knopfs");
em.persist(family);
for (int i = 0; i < 40; i++) {
Person person = new Person();
person.setFirstName("Jim_" + i);
person.setLastName("Knopf_" + i);
em.persist(person);
// now persists the family person relationship
family.getMembers().add(person);
em.persist(person);
em.persist(family);
}
}
// Commit the transaction, which will cause the entity to
// be stored in the database
em.getTransaction().commit();
// It is always good practice to close the EntityManager so that
// resources are conserved.
em.close();
}
@Test
public void checkAvailablePeople() {
// now lets check the database and see if the created entries are there
// create a fresh, new EntityManager
EntityManager em = factory.createEntityManager();
// Perform a simple query for all the Message entities
Query q = em.createQuery("select m from Person m");
// We should have 40 Persons in the database
assertTrue(q.getResultList().size() == 40);
em.close();
}
@Test
public void checkFamily() {
EntityManager em = factory.createEntityManager();
// Go through each of the entities and print out each of their
// messages, as well as the date on which it was created
Query q = em.createQuery("select f from Family f");
// We should have one family with 40 persons
assertTrue(q.getResultList().size() == 1);
assertTrue(((Family) q.getSingleResult()).getMembers().size() == 40);
em.close();
}
@Test(expected = javax.persistence.NoResultException.class)
public void deletePerson() {
EntityManager em = factory.createEntityManager();
// Begin a new local transaction so that we can persist a new entity
em.getTransaction().begin();
Query q = em
.createQuery("SELECT p FROM Person p WHERE p.firstName = :firstName AND p.lastName = :lastName");
q.setParameter("firstName", "Jim_1");
q.setParameter("lastName", "Knopf_!");
Person user = (Person) q.getSingleResult();
em.remove(user);
em.getTransaction().commit();
Person person = (Person) q.getSingleResult();
// Begin a new local transaction so that we can persist a new entity
em.close();
}
}
The project should now look like the following.
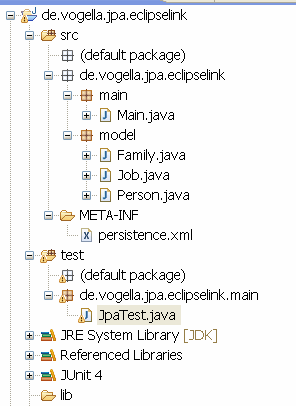
You should be able to run the JUnit tests successfully.
7. Links and Literature
7.1. Java Persistence API Resources
If you need more assistance we offer Online Training and Onsite training as well as consulting