Eclipse Microsoft Integration. This article will demonstrate how Eclipse SWT can be used to integrate / use Microsoft applications. Eclipse is using the SWT GUI framework. SWT does allow to integrated Microsoft application via OLE (Object Linking and Embedding).Microsoft Outlook, Microsoft Excel and the File explorer are used as examples. The examples used are based on Eclipse RCP as this makes it easy to demonstrate the usage but work with stand-alone Java application (which are using SWT). This article assumes that you are already familiar with using the Eclipse IDE and with developing simple Eclipse RCP applications.
1. Microsoft Object Linking and Embedding
Windows applications use OLE (Object Linking and Embedding) to allow applications to control other application objects. The following will show how to do this using a few examples. Obviously the following examples assume that you are running on Microsoft Windows and have the application which is discussed installed.
2. Microsoft Outlook with Eclipse
This example assumes you running an MS operating system and that you have a version of Outlook installed.
2.1. Create Project
Create a new project "de.vogella.microsoft.outlook". See Eclipse RCP for details. Use the "Hello RCP " as a template.
2.2. Create a command
Using extensions create the command "de.vogella.microsoft.outlook.sendEmail" and add it to the menu. See Eclipse command for details. Program the default handler "de.vogella.microsoft.outlook.handler.SendEmail"
The following code creates and opens the email for the user. It assumes that you have a file c:\temp\test.txt which will be attached to the email.
package de.vogella.microsoft.outlook.handler;
import java.io.File;
import org.eclipse.core.commands.AbstractHandler;
import org.eclipse.core.commands.ExecutionEvent;
import org.eclipse.core.commands.ExecutionException;
import org.eclipse.jface.dialogs.MessageDialog;
import org.eclipse.swt.SWT;
import org.eclipse.swt.ole.win32.OLE;
import org.eclipse.swt.ole.win32.OleAutomation;
import org.eclipse.swt.ole.win32.OleClientSite;
import org.eclipse.swt.ole.win32.OleFrame;
import org.eclipse.swt.ole.win32.Variant;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class SendEmail extends AbstractHandler {
@Override
public Object execute(ExecutionEvent event) throws ExecutionException {
Display display = Display.getCurrent();
Shell shell = new Shell(display);
OleFrame frame = new OleFrame(shell, SWT.NONE);
// This should start outlook if it is not running yet
OleClientSite site = new OleClientSite(frame, SWT.NONE, "OVCtl.OVCtl");
site.doVerb(OLE.OLEIVERB_INPLACEACTIVATE);
// now get the outlook application
OleClientSite site2 = new OleClientSite(frame, SWT.NONE,
"Outlook.Application");
OleAutomation outlook = new OleAutomation(site2);
//
OleAutomation mail = invoke(outlook, "CreateItem", 0 /* Mail item */)
.getAutomation();
setProperty(mail, "To", "test@gmail.com"); /*
* Empty but could be
* predefined
*/
setProperty(mail, "Bcc", "test@gmail.com"); /*
* Empty but could be
* predefined
*/
setProperty(mail, "BodyFormat", 2 /* HTML */);
setProperty(mail, "Subject", "Top News for you");
setProperty(mail, "HtmlBody",
"<html>Hello<p>, please find some infos here.</html>");
File file = new File("c:/temp/test.txt");
if (file.exists()) {
OleAutomation attachments = getProperty(mail, "Attachments");
invoke(attachments, "Add", "c:/temp/test.txt");
} else {
MessageDialog
.openInformation(shell, "Info",
"Attachment File c:/temp/test.txt not found; will send email with attachment");
}
invoke(mail, "Display" /* or "Send" */);
return null;
}
private static OleAutomation getProperty(OleAutomation auto, String name) {
Variant varResult = auto.getProperty(property(auto, name));
if (varResult != null && varResult.getType() != OLE.VT_EMPTY) {
OleAutomation result = varResult.getAutomation();
varResult.dispose();
return result;
}
return null;
}
private static Variant invoke(OleAutomation auto, String command,
String value) {
return auto.invoke(property(auto, command),
new Variant[] { new Variant(value) });
}
private static Variant invoke(OleAutomation auto, String command) {
return auto.invoke(property(auto, command));
}
private static Variant invoke(OleAutomation auto, String command, int value) {
return auto.invoke(property(auto, command),
new Variant[] { new Variant(value) });
}
private static boolean setProperty(OleAutomation auto, String name,
String value) {
return auto.setProperty(property(auto, name), new Variant(value));
}
private static boolean setProperty(OleAutomation auto, String name,
int value) {
return auto.setProperty(property(auto, name), new Variant(value));
}
private static int property(OleAutomation auto, String name) {
return auto.getIDsOfNames(new String[] { name })[0];
}
}
If you now start the application and press the button an email should be prepared and shown to the user.

3. Microsoft Excel with Eclipse
3.1. Create Project
Eclipse RCP for details. Use the "RCP with a view" as a template. Run it and see that is working.
3.2. Change View code
Select View.java and replace the code with the following.
package exceltest;
import org.eclipse.swt.SWT;
import org.eclipse.swt.SWTError;
import org.eclipse.swt.ole.win32.OleClientSite;
import org.eclipse.swt.ole.win32.OleFrame;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.ui.part.ViewPart;
public class View extends ViewPart {
public static final String ID = "ExcelTest.view";
private OleClientSite site;
public View() {
}
@Override
public void createPartControl(Composite parent) {
try {
OleFrame frame = new OleFrame(parent, SWT.NONE);
site = new OleClientSite(frame, SWT.NONE, "Excel.Sheet");
} catch (SWTError e) {
System.out.println("Unable to open activeX control");
return;
}
}
@Override
public void setFocus() {
// Have to set the focus see https://bugs.eclipse.org/bugs/show_bug.cgi?id=207688
site.setFocus();
}
}
Start your application and excel should be displayed.
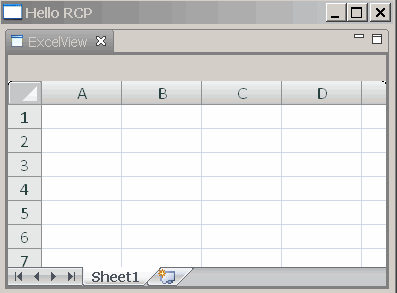
4. Microsoft File Explorer with Eclipse
Create a new project "de.vogella.microsoft.fileexplorer". See Eclipse RCP for details.
Add a view to the application and the perspective. To display the file explorer you can use the following code for your view.
package de.vogella.microsoft.fileexplorer;
import org.eclipse.swt.SWT;
import org.eclipse.swt.SWTError;
import org.eclipse.swt.ole.win32.OLE;
import org.eclipse.swt.ole.win32.OleAutomation;
import org.eclipse.swt.ole.win32.OleClientSite;
import org.eclipse.swt.ole.win32.OleFrame;
import org.eclipse.swt.ole.win32.Variant;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.ui.part.ViewPart;
public class FileExplorerView extends ViewPart {
private OleClientSite site;
static final int Navigate = 0x68;
@Override
public void createPartControl(Composite parent) {
try {
OleFrame frame = new OleFrame(parent, SWT.NONE);
site = new OleClientSite(frame, SWT.NONE, "Shell.Explorer.1");
site.doVerb(OLE.OLEIVERB_INPLACEACTIVATE);
OleAutomation auto = new OleAutomation(site);
auto.invoke(Navigate, new Variant[] { new Variant("c:\\temp") });
} catch (SWTError e) {
System.out.println("Unable to open activeX control");
return;
}
}
@Override
public void setFocus() {
site.setFocus();
}
}
5. Links and Literature
Nothing listed.
5.1. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting