This tutorial describes the usage of the fused location provider.
1. Android Location API
1.1. Determine the current geolocation
Most Android devices allow to determine the current geo location. This can be done via a GPS (Global Positioning System) module, via cell tower triangulation and via wifi networks.
Google Play provides the fused location provider to retrieve the device’s last known location.
1.2. Installation
To use the location manager make the Google play service available via your app build.gradle file.
dependencies {
compile 'com.google.android.gms:play-services:9.2.0'
compile 'com.google.android.gms:play-services-location:9.2.0'
}
Also specify the following required permission in your manifest.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
1.3. Using the LocationManager
Now you can access the last known location. The fuse location provider provides a new simple API. The following is an example activity which uses it.
1.4. Forward and reverse Geocoding
The
Geocoder
class allows to determine the geo-coordinates
(longitude, laditude)
for a given address and possible addresses for
given geo-coordinates.
This process is known as forward and reverse
geocoding. The
Geocoder
class uses an online Google service.
1.5. Security
If you want to access the GPS sensor, you need the
ACCESS_FINE_LOCATION
permission. Otherwise you need the
ACCESS_COARSE_LOCATION
permission.
1.6. Prompt the user to Enabled GPS
The user can decide if the GPS is enabled or not.
You can find out, if a LocationManager is enabled via the
isProviderEnabled()
method. If its not enabled you can send the user to the settings via
an
Intent
with the
Settings.ACTION_LOCATION_SOURCE_SETTINGS
action for the
android.provider.Settings
class.
LocationManager service = (LocationManager) getSystemService(LOCATION_SERVICE);
boolean enabled = service
.isProviderEnabled(LocationManager.GPS_PROVIDER);
// check if enabled and if not send user to the GSP settings
// Better solution would be to display a dialog and suggesting to
// go to the settings
if (!enabled) {
Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS);
startActivity(intent);
}
Typically you would open an
AlarmDialog
prompt the user
and if he wants to enable GPS or if the application
should be canceled.
You cannot enable the GPS directly in your code, the user has to do this.
2. Using GPS and setting the current location
2.1. Activating GPS on the emulator
You need to activate GPS on your test device. If you test on the
emulator and its not activated you
"null" if you try to use a
LocationManager
.
The Google Map activity should automatically activate the GPS device in the emulator but if you want to use the location manager directly you need to do this yourself. Currently their seems to be an issue with this.
Start Google Maps on the emulator and request the current geo-position, this will allow you to activate the GPS. Send new GPS coordinates to the Android emulator.
2.2. Setting the geoposition
You can use the "DDMS"
Perspective
of Eclipse to send your geoposition to the emulator or a connected
device. For open this
Perspective
select
.
In the Emulator Control part you can enter the geocoordinates and press the Send button.
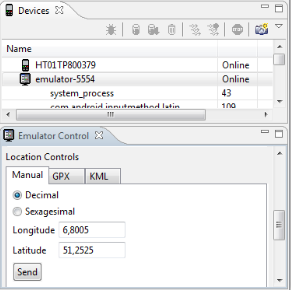
You can also set the geoposition the Android emulator via telnet. Open a console and connect to your device. The port number of your device can be seen in the title area of your emulator.
telnet localhost 5554
Set the position via the following command.
geo fix 13.24 52.31
3. Tutorial: Using the Android Location API
3.1. Create Project
Create a new project called de.vogella.android.locationapi.simple with the Activity called ShowLocationActivity.
This example will not use the Google Map therefore, it also runs on an Android device.
Change your layout file from the <filename class="directory">res/layout_ folder to the following code.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="40dip"
android:orientation="horizontal" >
<TextView
android:id="@+id/TextView01"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="10dip"
android:layout_marginRight="5dip"
android:text="Latitude: "
android:textSize="20dip" >
</TextView>
<TextView
android:id="@+id/TextView02"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="unknown"
android:textSize="20dip" >
</TextView>
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<TextView
android:id="@+id/TextView03"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="10dip"
android:layout_marginRight="5dip"
android:text="Longitute: "
android:textSize="20dip" >
</TextView>
<TextView
android:id="@+id/TextView04"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="unknown"
android:textSize="20dip" >
</TextView>
</LinearLayout>
</LinearLayout>
3.2. Add permissions
Add the following permissions to your application in your AndroidManifest.xml file
-
INTERNET
-
ACCESS_FINE_LOCATION
-
ACCESS_COARSE_LOCATION
3.3. Activity
Change
ShowLocationActivity
to the following. It queries the location manager and display the
queried
values in
the activity.
package de.vogella.android.locationsapi.simple;
import android.app.Activity;
import android.content.Context;
import android.location.Criteria;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.widget.TextView;
import android.widget.Toast;
public class ShowLocationActivity extends Activity implements LocationListener {
private TextView latituteField;
private TextView longitudeField;
private LocationManager locationManager;
private String provider;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
latituteField = (TextView) findViewById(R.id.TextView02);
longitudeField = (TextView) findViewById(R.id.TextView04);
// Get the location manager
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
// Define the criteria how to select the locatioin provider -> use
// default
Criteria criteria = new Criteria();
provider = locationManager.getBestProvider(criteria, false);
Location location = locationManager.getLastKnownLocation(provider);
// Initialize the location fields
if (location != null) {
System.out.println("Provider " + provider + " has been selected.");
onLocationChanged(location);
} else {
latituteField.setText("Location not available");
longitudeField.setText("Location not available");
}
}
/* Request updates at startup */
@Override
protected void onResume() {
super.onResume();
locationManager.requestLocationUpdates(provider, 400, 1, this);
}
/* Remove the locationlistener updates when Activity is paused */
@Override
protected void onPause() {
super.onPause();
locationManager.removeUpdates(this);
}
@Override
public void onLocationChanged(Location location) {
int lat = (int) (location.getLatitude());
int lng = (int) (location.getLongitude());
latituteField.setText(String.valueOf(lat));
longitudeField.setText(String.valueOf(lng));
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
@Override
public void onProviderEnabled(String provider) {
Toast.makeText(this, "Enabled new provider " + provider,
Toast.LENGTH_SHORT).show();
}
@Override
public void onProviderDisabled(String provider) {
Toast.makeText(this, "Disabled provider " + provider,
Toast.LENGTH_SHORT).show();
}
}
3.4. Run and Test
If you using the emulator send some geo-coordinates to your device. These geo-coordinate should be displayed as soon as you press the button.
4. Links and Literature
4.2. vogella Java example code
If you need more assistance we offer Online Training and Onsite training as well as consulting